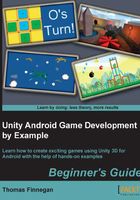
GUI Skins and GUI Styles
GUI Styles are how we change the look and feel of GUI elements, buttons, and labels in Unity. A GUI Skin contains several GUI Styles and allows us to change the look of the entire GUI without explicitly defining GUI Styles for each element. To create a GUI Skin, right-click in the Project window of the Unity Editor, just as with creating a new script. Go to Create but, instead of selecting Script, go to the bottom and select GUI skin. Selecting this option will create the new GUI Skin and let us name it to GameSkin
. By looking at our GameSkin
in the Inspector window, you can see what we have to work with.

- At the top is a Font attribute. By importing font files to your project and placing one here, you can change the default font used by text in the whole game.
- Under that is a long list of GUI elements, including our good friends Button and Label. These are all GUI Styles and coincide with the GUI functions that we use to draw things on screen. For example, unless otherwise specified, when we use the
Button
function, it will draw using the Button GUI Style. - Following the list of GUI elements is a Custom Styles attribute. This is where we can put any extra styles that we want to use. Of our dozen buttons, perhaps we want one to have red text. That GUI Style would go here.
- At the bottom is a Settings attribute. By expanding it, we can see it is fairly short. It includes options for whether or not multiclicks can be used for selection, what color of cursor and how fast it should flash when in a text field, and what color the highlight on selected words should be. The defaults here are just fine. Unless there is a very specific look or need, these values can be ignored.
Now, let us go over what it takes to be a GUI Style. Expand the Button GUI Style from our GameSkin
example. No matter what the GUI Style is used for, they all are made up the same. It may look like there are many attributes that make up a GUI Style, but most of them are nearly identical, making it much simpler.

- The first attribute is fairly straightforward, but perhaps the most important. Name is what Unity uses to find GUI Styles and apply them to GUI elements. It lets us know what the style is supposed to be; however, if there is a typo between it and the code, you will never see your style in the game.
- The next several groups of values describe how the GUI element should look when in a particular state. This is where the bulk of your styling will go. The primary states of any element are Normal, Hover, Active, and Focused. Secondary to these are On Normal, On Hover, On Active, and On Focused. These secondary states only occur as the GUI element transfers into the corresponding primary state. Not every GUI element makes use of every state, and you have the ability to control which states an element can go into, but we will discuss that a little later. Let's see in detail how these states work:
- Normal: This is the default state of any GUI element. It is always used and occurs any time the element is not being interacted with.
- Hover: This state is used primarily by buttons and other clickable elements. When your mouse is on top of a GUI element, it will enter this state, if it can. However, since the focus of this book is touch screens, we do not have a mouse to really concern ourselves with. So, we will not be using this state.
- Active: This has to be the second most important state. An element enters this state when it is activated. For example, when one presses a button, it is active. By clicking on or touching a button, it enters the Active state. All of the GUI elements that can be interacted with use this state.
- Focused: This is a rarely used state. In terms of Unity's GUI, focused means having keyboard control. The only element that uses it by default is the Text Field.
- If you were to expand any of the states, you would see that it has two attributes, Background and Text Color. The Background attribute is a texture. It can be any texture in your game. The Text Color attribute is simply the color of any text that appears in the GUI element. Except for the Normal state, if a state does not have a background texture, it will not be used. This can be both good and annoying. If we do not want our buttons to show that they have been hovered over, simply remove the texture from the Hover state's Background attribute. It becomes annoying when we want a GUI element that does not have a background image of its own, but we do want the text to change color between states. How do we make use of the active state, but not use a texture for the background? The answer is that we create a blank image, but it is not quite as simple as saving off a 100 percent transparent PNG and using that. The GUI Style is too smart for that. It detects that the image is completely blank, making it no different than if there was no image. And so, the state still is not used. To get around this, create a small, blank PNG image, but take a single pixel and make it 90 percent transparent white. This might seem like a hack solution, but it is, unfortunately, the only way. At such a low transparency, we can't detect the pixel; though it is not actually clear. However, Unity sees that there is a slightly white pixel that must be drawn and does so.
- Now, you might be thinking, that's stupid. I'm just going to create images of all of my buttons and not worry about the text. It is indeed stupid but the response to that is, what if you need to slightly change the text of a button? Or perhaps the text on the button is dynamic based on the player's name. In nearly every project I have been a part of there has been a need to create the not quite blank image.
- Below the GUI element's states are Border, Margin, Padding, and Overflow. These attributes control how an element interacts with its background images and contained text. Inside each you will find Left, Right, Top, and Bottom values. Since every element is drawn as a rectangle, these correspond to each side of the said rectangle. They are defined in pixels, just like our GUI space. Let's see all these attributes in detail as follows:
- Border: This lets us define how many pixels from each side should not be stretched. When defining a GUI element, the background is normally stretched evenly across the space occupied. If you were to create a blue box with red edging and rounded corners, these values would keep your edges and corners regular while still stretching the blue on the inside.
- Margin: This is only used by Unity's automatic GUI layout system named GUILayout. It is how much extra space should be around the outside of the element.
- Padding: This is the space between the borders of an element and the text that it contains. If you want the text of a button left-justified but in slightly, you should use Padding.
- Overflow: This defines an extra space for your background image. When creating our buttons, we defined a
Rect
class for how much space the button takes up. If we are to useOverflow
, the button itself would be where theRect
class is, but the background would extend beyond each edge as dictated by the values. This would be useful for buttons with a shadow or glow around them.
- The next several values have to do with the text in an element. The Font attribute is a font that is used specifically by this style. If this value is left empty, the font from the GUI Skin is used. Font Size is how big the letters of the text should be. This works just like your favorite word processor, except that a value of zero means to use the default font size defined in the font object. Font Style also works like your word processor. It lets you choose between Normal, Bold, and Italic text. This only makes a difference if it is supported by your chosen font.
- Alignment defines where to justify the text in the GUI element. Imagine splitting your element into a 3 x 3 grid. Alignment is the same as the position of the grid.
- Word Wrap defines whether or not text should split into multiple lines if it is too long. It again works on the same principle as your word processor. If checked and the line of text would extend beyond the sides of the GUI element, the text is split into as many lines as necessary to keep it within the bounds.
- Rich Text is a fairly new and interesting feature of GUI Styles. It allows us to use HTML style markup to control text. You could put the tags
<b>
and</b>
around a word in your Label's text, and instead of writing those tags, Unity will make the words in between bold. We can make use of the bold, italics, size, and color tags. This allows for selectively making parts of our text bold or italics. We can make certain words larger or smaller. And, the color of any part of the text can be altered using hexadecimal values. - Text Clipping became weird in the recent updates. It used to be a nice drop-down list of values, but now it is an integer field. Either way, it still serves its function. If the text extends beyond the edges of the GUI element, this attribute dictates what to do. A value of zero means don't clip the text, let it extend beyond the edges. Any value that is not zero will cause the text to be clipped. Any text that extends beyond the borders will simply not be drawn.
- Image Position is used in conjunction with GUIContent. GUIContent is a way of passing GUI elements the text, an icon image, and a tool tip. Image Position describes how the image and text interact. The image can either go to the left of the text or above. Or, we can choose to only use either the image or the text. Since tool tips aren't really useful in a touch environment, GUIContent is of limited use to us. For that reason, we will not be using it extensively, if at all.
- Content Offset adjusts anything contained inside the GUI element by the values provided. If all of your text is normally centered in your button, this will allow you to move it slightly to the right and up. It is an aesthetic thing, for when you need a very specific look.
- Fixed Width and Fixed Height provides approximately the same function. If any value other than zero is provided for these attributes, they will override the corresponding values in the
Rect
class used for the GUI element. So, if you wanted buttons to always be one hundred pixels wide, no matter where they are in the game, you could set Fixed Width to one hundred and they will do just that. - Stretch Width and Stretch Height also serves about the same function. They are used by GUILayout for automatic placement of GUI elements. It pretty much gives the system permission to make elements wider/skinnier and taller/shorter, respectively, in order to satisfy its conditions for a better layout. The way GUILayout arranges elements is not always the best. It is good, if you need something up quick. But it gets complicated if you want any sort of deeper control.