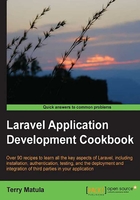
Creating a custom error message
Laravel has built-in error messages if a validation fails, but we may want to customize those messages to make our application unique. This recipe shows a few different ways to create custom error messages.
Getting ready
For this recipe, we just need a standard installation of Laravel.
How to do it...
To complete this recipe, follow these steps:
- Create a route in
routes.php
to hold the form:Route::get('myform', function() { return View::make('myform'); });
- Create a view named
myform.php
and add a form:<h1>User Info</h1> <?php $messages = $errors->all ('<p style="color:red">:message</p>') ?> <?php foreach ($messages as $msg) { echo $msg; } ?> <?= Form::open() ?> <?= Form::label('email', 'Email') ?> <?= Form::text('email', Input::old('email')) ?> <br> <?= Form::label('username', 'Username') ?> <?= Form::text('username', Input::old('username')) ?> <br> <?= Form::label('password', 'Password') ?> <?= Form::password('password') ?> <br> <?= Form::submit('Send it!') ?> <?= Form::close() ?>
- Create a route that handles our POST data and validates it:
Route::post('myform', array('before' => 'csrf', function() { $rules = array( 'email' => 'required|email|min:6', 'username' => 'required|min:6', 'password' => 'required' ); $messages = array( 'min' => 'Way too short! The :attribute must be atleast :min characters in length.', 'username.required' => 'We really, really need aUsername.' ); $validation = Validator::make(Input::all(), $rules,$messages); if ($validation->fails()) { return Redirect::to('myform')->withErrors($validation)->withInput(); } return Redirect::to('myresults')->withInput(); }));
- Open the file
app/lang/en/validation.php
, whereen
is the default language of the app. In our case, we're using English. At the bottom of the file, update theattributes
array as the following:'attributes' => array( 'password' => 'Super Secret Password (shhhh!)' ),
- Create a route to handle a successful form submission:
Route::get('myresults', function() { return dd(Input::old()); });
How it works...
We first create a fairly simple form, and since we aren't passing any parameters to Form::open()
, it will POST the data to the same URL. We then create a route to accept the POST
data and validate it. As a best practice, we're also adding in the csrf
filter before our post
route. This will provide some extra security against cross-site request frogeries.
The first variable we set in our post
route will hold our rules. The next variable will hold any custom messages we want to use if there's an error. There are a few different ways to set the message.
The first message to customize is for min
size. In this case, it will display the same message for any validation errors where there's a min
rule. We can use :attribute
and :min
to hold the form field name and minimum size when the error is displayed.
Our second message is used only for a specific form field and for a specific validation rule. We put the form field name first, followed by a period, and then the rule. Here, we are checking whether the username is required and setting the error message.
Our third message is set in the language file for validations. In the attributes
array, we can set any of our form field names to display any custom text we'd like. Also, if we decide to customize a particular error message across the entire application, we can alter the default message at the top of this file.
There's more...
If we look in the app/lang
directory, we see quite a few translations that are already part of Laravel. If our application is localized, we can set custom validation error messages in any language we choose.
See also
- The Creating a simple form recipe