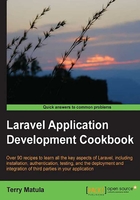
Setting up and configuring the Auth library
To use Laravel's authentication system, we need to make sure it's set up correctly. In this recipe, we'll see a common way to accomplish the setup.
Getting ready
To set up the authentication, we just need to have Laravel installed and a MySQL instance running.
How to do it...
To complete this recipe, follow these steps:
- Go into your
app/config/session.php
config file and make sure it's set to usenative
:'driver' => 'native'
- The
app/config/auth.php
config file defaults should be fine but make sure they are set as follows:'driver' => 'eloquent', 'model' => 'User', 'table' => 'users',
- In MySQL, create a database named as
authapp
and make sure the settings are correct in theapp/config/database.php
config file. The following are the settings that we'll be using:'default' => 'mysql', 'connections' => array( 'mysql' => array( 'driver' => 'mysql', 'host' => 'localhost', 'database' => 'authapp', 'username' => 'root', 'password' => '', 'charset' => 'utf8', 'prefix' => '', ), ),
- We'll set up our
Users
table using migrations and the Schema builder with the Artisan command line, so we need to create our migrations table:php artisan migrate:install
- Create the migration for our
Users
table:php artisan migrate:make create_users_table
- In the
app/database/migrations
directory, there will be a new file that has the date followed bycreate_users_table.php
as the filename. In that file, we create our table:<?php use Illuminate\Database\Migrations\Migration; class CreateUsersTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('users', function($table) { $table->increments('id'); $table->string('email'); $table->string('password', 64); $table->string('name'); $table->boolean('admin'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::drop('users'); } }
- Run the migration in Artisan to create our table and everything should be set up:
php artisan migrate
How it works...
Authentication uses sessions to store user information, so we first need to make sure our Sessions are configured correctly. There are various ways to store sessions, including using the database or Redis, but for our purpose we'll just use the native
driver, which leverages Symfony's native session driver.
When setting up the auth configuration, we'll be using the Eloquent ORM as our driver, an e-mail address as our username, and the model will be User. Laravel ships with a default User model and it works very well out of the box, so we'll use it. For the sake of simplicity, we'll stick with the default configuration of the table name, a pluralized version of the model class name, but we could customize it if we wanted.
Once we make sure our database configuration is set, we can use Artisan to create our migrations. In our migration, we'll create our user's table, and store the e-mail address, password, a name, and a boolean field to store whether the user is an admin or not. Once that's complete, we run the migration, and our database will be set up to build our authentication system.