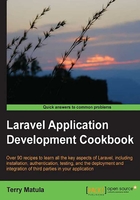
Retrieving and updating user info after logging in
After a user is logged in, we will need to get the information we have about him/her. In this recipe, we'll see how to get that information.
Getting ready
We will be using the code created in the Setting up and configuring the Auth library and Creating an authentication system recipes as the basis for this recipe.
How to do it...
To complete this recipe, follow these steps:
- Update the profile route with this code:
Route::get('profile', function() { if (Auth::check()) { return View::make('profile')->with('user',Auth::user()); } else { return Redirect::to('login')->with('login_error','You must login first.'); } });
- Create our profile view in the
app/views
directory by creating a file named asprofile.php
:<?php echo Session::get('notify') ? "<p style='color: green'>" . Session::get('notify') . "</p>" : "" ?> <h1>Welcome <?php echo $user->name ?></h1> <p>Your email: <?php echo $user->email ?></p> <p>Your account was created on: <?php echo $user ->created_at ?></p> <p><a href="<?= URL::to('profile-edit') ?>">Edit your information</a></p>
- Make a route to hold our form to edit the information:
Route::get('profile-edit', function() { if (Auth::check()) { $user = Input::old() ? (object) Input::old() :Auth::user(); return View::make('profile_edit')->with('user',$user); } });
- Create a view for our edit form:
<h2>Edit User Info</h2> <?php $messages = $errors->all('<p style="color:red">:message</p>') ?> <?php foreach ($messages as $msg): ?> <?= $msg ?> <?php endforeach; ?> <?= Form::open() ?> <?= Form::label('email', 'Email address: ') ?> <?= Form::text('email', $user->email) ?> <br> <?= Form::label('password', 'Password: ') ?> <?= Form::password('password') ?> <br> <?= Form::label('password_confirm', 'Retype Password: ') ?> <?= Form::password('password_confirm') ?> <br> <?= Form::label('name', 'Name: ') ?> <?= Form::text('name', $user->name) ?> <br> <?= Form::submit('Update!') ?> <?= Form::close() ?>
- Make a route to process the form:
Route::post('profile-edit', function() { $rules = array( 'email' => 'required|email', 'password' => 'same:password_confirm', 'name' => 'required' ); $validation = Validator::make(Input::all(), $rules); if ($validation->fails()) { return Redirect::to('profile-edit')->withErrors($validation)->withInput(); } $user = User::find(Auth::user()->id); $user->email = Input::get('email'); if (Input::get('password')) { $user->password = Hash::make(Input::get('password')); } $user->name = Input::get('name'); if ($user->save()) { return Redirect::to('profile')->with('notify','Information updated'); } return Redirect::to('profile-edit')->withInput(); });
How it works...
To get our user's information and allow him/her to update it, we start by reworking on our profile route. We create a profile view and pass Auth::user()
to it in the variable $user
. Then, in the view file, we simply echo out any of the information we collected. We're also creating a link to a page where the user can edit his/her information.
Our profile edit page first checks to make sure the user is logged in. If so, we want to populate the $user
variable. Since we'll redisplay the form if there is a validation error, we first check if there's anything in Input::old()
. If not, this is probably a new visit to the page, so we just use Auth::user()
. If Input::old()
is being used, we'll recast it as an object, since it's normally an array, and use that in our $user
variable.
Our edit view form is very similar to our registration form, except that, if we're logged in, the form is already populated.
When the form is submitted, it is run through some validation. If everything is valid, we need to get the User from the database, using User::find()
and the user ID that's stored in Auth::user()
. We then add our form input to the user object. With the password field, if it was left empty, we can assume that the user didn't want to change it. So we'll only update the password if something was already entered.
Finally, we save the user information and redirect him/her back to the profile page.
There's more...
The e-mail value in our database will probably need to be unique. For this recipe, we might want to do a quick check of the user's table, and make sure the e-mail address being updated isn't used somewhere else.
See also
- The Creating an authentication system recipe