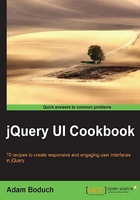
Resizable content sections
Resizable content sections allow the user to adjust the height by dragging the bottom of the section. This is a nice alternative having to rely on the heightStyle
property. Thus, if each section of the accordion can be adjusted by the user, they have the freedom to tailor the accordion layout. For example, if the accordion has a tall section, with wasted space at the bottom, the user might choose to shrink the height of that section to gain a better view of the accordion, and other components of the UI for that matter.
How to do it...
We'll extend the default accordion's _create()
method by making each content's div
within the accordion resizable using the resizable interaction widget.
( function( $, undefined ) { $.widget( "ab.accordion", $.ui.accordion, { _create: function () { this._super( "_create" ); this.headers.next() .resizable( { handles: "s" } ) .css( "overflow", "hidden" ); }, _destroy: function () { this._super( "_destroy" ); this.headers.next() .resizable( "destroy" ) .css( "overflow", "" ); } }); })( jQuery ); $( function() { $( "#accordion" ).accordion(); });
You'll see something similar to the following. Notice that the second section has been dragged down and has the resize mouse cursor.

How it works...
Our new version of the _create()
method works by first invoking the default accordion's _create()
method. Once that completes, we find all content sections of the accordion and apply the resizable()
widget. You'll notice, too, that we've told the resizable widget to only show a south
handle. This means that the user will only be able to drag any given content section of the accordion up or down, using the cursor at the bottom of the section.
This specialization of an accordion also provides a new implementation of the _delete()
method. Once again, we're calling the original accordion's _delete()
, after which we're cleaning up the new resizable components we added. This includes removing the overflow
CSS property.
There's more...
We can extend our resizable behavior within the accordion by providing a means to turn it off. We'll add a simple resizable
option to the accordion that checks whether or not to make the accordion sections resizable.
(function( $, undefined ) { $.widget( "ab.accordion", $.ui.accordion, { options: { resizable: true }, _create: function () { this._super( "_create" ); if ( !this.options.resizable ) { return; } this.headers.next() .resizable( { handles: "s" } ) .css( "overflow", "hidden" ); }, _destroy: function () { this._super( "_destroy" ); if ( !this.options.resizable ) { return; } this.headers.next() .resizable( "destroy" ) .css( "overflow", "" ); }, }); })( jQuery ); $(function() { $( "#accordion" ).accordion( { resizable: false } ); });