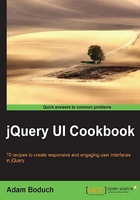
Using multiple data sources
Sometimes, an autocomplete widget doesn't map directly to one data source. Take video for instance. Imagine the user needs to pick a video, but the two data sources are DVD and Blu-ray. If we were to use autocomplete to select a video, we would need a way to assign multiple data sources. Furthermore, the mechanism would need to be extensible enough to support adding more data sources, especially since there is a new video format born every other year.
How to do it...
The default implementation of the autocomplete widget is expecting a single data source – an array or an API endpoint string. We'll give the widget a new sources
option to allow for this behavior. This is how we'll extend autocomplete and create an instance of the widget that has two video data sources – one for DVDs, and one for Blu-ray discs.
( function( $, undefined ) { $.widget( "ab.autocomplete", $.ui.autocomplete, { options: { sources: [] }, _create: function() { var sources = this.options.sources; if ( sources.length ) { this.options.source = function ( request, response ) { var merged = [], filter = $.ui.autocomplete.filter; $.each( sources, function ( index, value ) { $.merge( merged, value ); }); response( filter( merged, request.term ) ); }; } this._super( "_create" ); }, _destroy: function() { this._super( "_destroy" ); } }); })( jQuery ); $( function() { var s1 = [ "DVD 1", "DVD 2", "DVD 3" ], s2 = [ "Blu-ray 1", "Blu-ray 2", "Blu-ray 3" ]; $( "#autocomplete" ).autocomplete({ sources: [s1, s2] }); });

As you can see, if you were to start searching for the video 1
, you'd get versions from each data source in the drop-down menu.
How it works...
Rather than merging our two data sources into one before it gets passed to the autocomplete, we're extending the capabilities of the widget to handle that task for us. In particular, we've added a new sources
option that can accept several arrays. In the example, we're passing both the DVD and the Blu-ray sources to our widget.
Our customized version of _create()
checks to see if multiple sources have been supplied by checking the length of the sources
option. If there are multiple data sources, we use the merge()
jQuery utility function to create a new array and apply the filter()
function to it. A good feature of this approach is that it doesn't care how many data sources there are—we could pass a few more to our implementation down the road should the application require it. The merging of these data sources is encapsulated behind the widget.