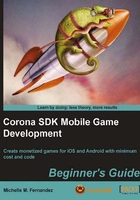
Valuable variables
Like in many scripting languages, Lua has variables. You can think of it as something that stores values. When you apply a value to a variable, you can refer to it using the same variable.
An application consists of statements and variables. Statements provide instructions on what operations and computations need to be done. Variables store the values of these computations. Setting a value into a variable is called an assignment.
Lua uses three kinds of variables: global, local, and table fields.
Global variables
A global variable can be accessed in every scope and can be modified from anywhere. The term scope is used to describe the area in which a set of variables live. You don't have to declare a global variable. It is created as soon as you assign a value to it.
myVariable = 10 print( myVariable ) -- prints the number 10
Local variables
A local variable is accessed from a local scope and usually called from a function or block of code. When we create a block, we are creating a scope in which variables can live or a list of statements, which are executed sequentially. When referencing a variable, Lua must find the variable. Localizing variables helps speed up the look-up process and improves the performance of your code. By using the local statement, it declares a local variable.
local i = 5 -- local variable
The following is how to declare a local variable in a block:
x = 10 -- global 'x' variable local i = 1 while i <= 10 do local x = i * 2 -- a local 'x' variable for the while block print( x ) -- 2, 4, 6, 8, 10 ... 20 i = i + 1 end print( x ) -- prints 10 from global x
Table fields (properties)
Table fields are elements of the table themselves. Arrays can be indexed with numbers and strings or any value pertaining to Lua except nil. You index into the array to assign the values to a field using integers. When the index is a string, the field is known as a property. All properties can be accessed using the dot operator (x.y
) or a string (x["y"]
) to index into a table. The result is the same.
x = { y="Monday" } -- create table print( x.y ) -- "Monday" z = "Tuesday" -- assign a new value to property "Tuesday" print( z ) -- "Tuesday" x.z = 20 -- create a new property print( x.z ) -- 20 print( x["z"] ) -- 20
More information relating to tables will be discussed later in the section called Tables.
You may have noticed the additional text on certain lines of code in the preceding examples. Those are what you call comments. Comments begin anywhere with a double hyphen --
, except inside a string. They run until the end of the line. Block comments are available as well. A common trick to comment out a block is to surround it with --[[
.
Here is how to comment one line:
a = 2
--print(a) -- 2
The following is a block comment:
--[[ k = 50 print(k) -- 50 ]]--
Assignment conventions
There are rules for variable names. A variable starts with a letter or an underscore. It can't contain anything other than letters, underscores, or digits. It also can't be one of the following words reserved by Lua:
The following are valid variables:
x
X
ABC
_abc
test_01
myGroup
The following are invalid variables:
function
my-variable
123