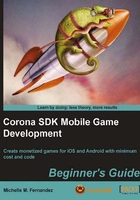
Time for action – getting our hands full of strings
We're starting to familiarize ourselves with several blocks of code and how they interact with each other. Let's see what happens when we add in some expressions using strings and how different they are in comparison to just regular strings you print out in the terminal.
- Create a new project folder on your desktop and name it
Working With Strings
. - Make a new
main.lua
file in your text editor and save it in your folder. - Type the following lines (Do not include the line numbers in the code, it is only used for line reference):
1 print("This is a string!") -- This is a string! 2 print("15" + 1) -- Returns the value 16
- Add in the following variables. Notice that it uses the same variable name:
3 myVar = 28 4 print(myVar) -- Returns 28 5 myVar = "twenty-eight" 6 print(myVar) -- Returns twenty-eight
- Let's add in more variables with some string values and compare them using different operators.
7 Name1, Phone = "John Doe", "123-456-7890" 8 Name2 = "John Doe" 9 print(Name1, Phone) -- John Doe 123-456-7890 10 print(Name1 == Phone) -- false 11 print(Name1 <= Phone) -- false 12 print(Name1 == Name2) -- true
- Save your script and launch your project in Corona. Observe the results in the terminal window.
This is a string! 16 28 twenty-eight John Doe 123-456-7890 false false true
What just happened?
You can see that line 1 is just a plain string with characters printed out. In line 2, notice that the number 15 is inside the string and then added to the number 1 which is outside the string. Lua provides automatic conversions between number and strings at runtime. Numeric operations applied to a string will try to convert the string to a number.
When working with variables, you can use the same one and have them contain a string and a number at different times as in lines 3 and 5 ( myVar = 28
and myVar = "twenty-eight"
).
In the last chunk of code (lines 7-12), we compared different variable names using relational operators. First, we printed the strings of Name1
and Phone
. The next lines following the comparison between Name1
, Name2
, and Phone
. When two strings have the same characters in the exact order, then they are considered as the same string and are equal to each other. When you look at print(Name1 == Phone)
and print(Name1 <= Phone)
, the characters do not correlate with each other so they return false
. In print(Name1 == Name2)
, both variables contain the same characters and therefore returns true
.
Have a go hero – pulling some more strings
Strings are pretty simple to work with since they are just sequences of characters. Try making your own expressions similar to the preceding example with the following modifications:
- Create some variables with numerical values and another set of variables with numerical string values. Use relational operators to compare the values and then print out the results.
- Use the concatenation operator and combine several strings or numbers together and space them out equally. Print out the result in the terminal window.