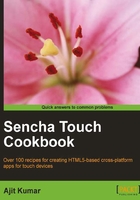
Setting up the Android-based development environment
This recipe describes the detailed steps we shall follow to set up the environment for the Android-based development. The steps do not include setting up the production environment, which are detailed in a different recipe.
Getting ready
Before you begin, check that JDK is installed and the following environment variables are set correctly:
JAVA_HOME
PATH
How to do it...
Carry out the following steps:
- Download and install Android SDK from the following URL: http://developer.android.com/sdk/index.html.
- Download and install Eclipse ADT Plugin from the following URL:http://developer.android.com/sdk/eclipse-adt.html#installing.
- Download and install PhoneGap from http://www.phonegap.com.
- Launch Eclipse, click on the File menu, and select New | Android Project. Fill in the details, as shown in the following screenshot, and click on the Finish button:
- In the root directory of the project, create two new directories:
libs
: To keep the third party jar that we will be using. In this case, we will keep the PhoneGap jar in itassets/www
: This is the default folder the SDK expects to contain the complete set of JS, CSS, and HTML files
- Copy
phonegap.1.0.0.js
from your PhoneGap downloaded earlier toassets/www
. - Copy
phonegap.1.0.0.jar
from your PhoneGap downloaded earlier tolibs
. - Copy the
xml
folder from theAndroid
folder of your PhoneGap downloaded earlier to theres
folder. - Make the following changes to
App.java
, found in thesrc
folder in Eclipse:- Change the class extend from
Activity
toDroidGap
- Replace the
setContentView()
line withsuper.loadUrl("file:///android_asset/www/index.html");
- Add
import com.phonegap.*;
- Change the class extend from
- Right-click on the
libs
folder and select Build Paths | Configure Build Paths. Then, in the Libraries tab, addphonegap-1.0.0.jar
to the Project. You may have to refresh the project (F5) once again. - Right-click on
AndroidManifest.xml
and select Open With | Text Editor. - Paste the following permissions under
versionName
:<supports-screensandroid:largeScreens="true"android:normalScreens="true"android:smallScreens="true"android:resizeable="true"android:anyDensity="true"/> <uses-permission android:name="android.permission.CAMERA" /> <uses-permission android:name="android.permission.VIBRATE" /> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_LOCATION_EXTRA_COMMANDS" /> <uses-permission android:name="android.permission.READ_PHONE_STATE" /> <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.RECEIVE_SMS" /> <uses-permission android:name="android.permission.RECORD_AUDIO" /> <uses-permission android:name="android.permission.MODIFY_AUDIO_SETTINGS" /> <uses-permission android:name="android.permission.READ_CONTACTS" /> <uses-permission android:name="android.permission.WRITE_CONTACTS" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" /> <uses-permission android:name="android.permission.ACCESS_NETWOvRK_STATE" />
Tip
Downloading the example code for this book
You can download the example code files for all Packt books you have purchased from your account at www.PacktPub.com. If you purchased this book elsewhere, you can visit http://www.PacktPub.com/support and register to have the files e-mailed directly to you.
- Add
android:configChanges="orientation|keyboardHidden"
to the activity tag inAndroidManifest
. - Move the
c:\sencha-touch
folder to theassets/www
directory. - Create and open a new file named
ch01_01.js
in theassets/www/ch01
directory. Paste the following code into it:Ext.setup({ onReady: function() { Ext.Msg.alert("INFO", "Welcome to the world of Sencha Touch!"); } });
- Now create and open a new file named
index.html
in theassets/www
directory and paste the following code into it:<!DOCTYPE HTML> <html> <head> <title>Yapps! - Your daily applications!</title> <link rel="stylesheet" href="sencha-touch/resources/css/sencha-touch.css" type="text/css"> <script type="text/javascript" charset="utf-8" src="phonegap.1.0.0.js"></script> <script type="text/javascript" charset="utf-8" src="sencha-touch/sencha-touch-debug.js"></script> <script type="text/javascript" charset="utf-8" src="ch01/ch01_01.js"></script> </head> <body></body> </html>
- Deploy to Simulator:
- Right-click on the project and go to Run As and click on Android Application
- Eclipse will ask you to select an appropriate AVD (Android Virtual Device). If there is not one, then you will need to create it. In order to create an AVD, follow these steps:
- In Eclipse, go to Window | Android SDK and AVD Manager
- Select Virtual Devices and click on the New button
- Enter your virtual device details, for example, the following screenshot shows the virtual device details for the Samsung Galaxy Ace running Android 2.2:
- Deploy to Device:
- Make sure USB debugging is enabled on your device and plug it into your system. You may enable it by going to Settings | Applications | Development
- Right-click on the project, go to Run As, and click on Android Application. This will launch the Android Device Chooser window
- Select the device and click on the OK button
With the preceding steps, you will be able to develop and test your application.
How it works...
In steps 1 through 3, we downloaded and installed Android SDK, its Eclipse plugin, and PhoneGap, which are required for the development of the Android-based application. The SDK contains the Android platform-specific files, an Android emulator, and various other tools required for the packaging, deployment, and running of Android-based applications. The ADT plugin for Eclipse allows us to create Android-based applications, build, test, and deploy them using Eclipse.
In step 4, we created an Android 2.2 project by using the ADT plugin.
In steps 5 through 12, we created the required folders and kept the files in those folders, and updated some of the files to make this Android project a PhoneGap-based Android project. In step 5, we created two folders: libs
and assets\www
. The libs
folder is where the PhoneGap and other third party libraries (JAR files) need to be kept. In our case, we only had to put in the PhoneGap JAR file (step 7). This JAR contains the PhoneGap implementation, which takes care of packaging the application for the target device (in this case, Android). The www
folder is where the complete application code needs to be kept. PhoneGap will use this folder to create the deployment package.
In step 6, we copied the PhoneGap's JavaScript file which contains the implementation of the PhoneGap APIs. You will do this if you intend to use the PhoneGap APIs in your application (for example, to get the contacts list in your application).
Note
For this book, this is an optional step. However, interested readers may find details about the API at the following URL: http://docs.phonegap.com/en/1.0.0/index.html.
In steps 8 and 9, we added the PhoneGap JAR file to our project's Build Path, so that it becomes available for the application development (and takes care of the compilation errors).
Then, in steps 10 through 13, we made changes to the manifest file to add the required application privileges, for example, access to the phone book, access to the phone status, and so on, when it is run on the Android 2.2 platform. You may learn more about the content of the manifest file and each of the elements that we added to it by referring to http://developer.android.com/guide/topics/manifest/manifest-intro.html.
In step 14, we moved the Sencha Touch library files to the www
folder, so that they are included in the package. This is required to run Touch-based applications.
In step 15, we created the ch01_01.js
JavaScript file, which contains the entry point for our Sencha Touch application. We have used the Ext.setup
API. The important property is onReady
, which is a function that Ext.setup
registers to invoke as soon as the document is ready.
In step 16, we modified the index.html
to include the Sencha Touch library related JavaScript: sencha-touch-debug.js
and CSS file: sencha-touch.css
, and our application specific JavaScript file: ch01_01.js
. The sencha-touch-debug.js
file is very useful during development as it contains the nicely formatted code which can be used to analyze the application errors during development. You also need to include the PhoneGap JS file, if you intend to use its APIs in your application. The order of inclusion of the JavaScript and CSS files is PhoneGap | Sencha Touch | Application specific files.
In step 17, we created an Android Virtual Device (an emulator), and deployed and tested the application on it.
Finally, in step 18 we deployed the application on a real Android 2.2 compatible device.