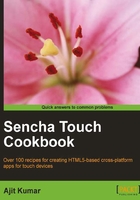
Getting your form ready with FormPanel
This recipe shows how to create a basic form using Sencha Touch and implement some of the behaviors such as submitting the form data, handling errors during the submission, and so on.
Getting ready
Make sure that you have set up your development environment by following the recipes outlined in Chapter 1, Gear up for the Journey.
How to do it...
Carry out the following steps:
- Create a
ch02
folder in the same folder where we had created thech01
folder. - Create and open a new file named
ch02_01.js
and paste the following code into it:Ext.setup({ onReady: function() { var form; //form and related fields config var formBase = { //enable vertical scrolling in case the form exceeds the page height scroll: 'vertical', url: 'http://localhost/test.php', items: [{//add a fieldset xtype: 'fieldset', title: 'Personal Info', instructions: 'Please enter the information above.', //apply the common settings to all the child items of the fieldset defaults: { required: true, //required field labelAlign: 'left', labelWidth: '40%' }, items: [ {//add a text field xtype: 'textfield', name : 'name', label: 'Name', useClearIcon: true,//shows the clear icon in the field when user types autoCapitalize : false }, {//add a password field xtype: 'passwordfield', name : 'password', label: 'Password', useClearIcon: false }, { xtype: 'passwordfield', name : 'reenter', label: 'Re-enter Password', useClearIcon: true }, {//add an email field xtype: 'emailfield', name : 'email', label: 'Email', placeHolder: 'you@sencha.com', useClearIcon: true }] } ], listeners : { //listener if the form is submitted, successfully submit : function(form, result){ console.log('success', Ext.toArray(arguments)); }, //listener if the form submission fails exception : function(form, result){ console.log('failure', Ext.toArray(arguments)); } }, //items docked to the bottom of the form dockedItems: [ { xtype: 'toolbar', dock: 'bottom', items: [ { text: 'Reset', handler: function() { form.reset(); //reset the fields } }, { text: 'Save', ui: 'confirm', handler: function() { //submit the form data to the url form.submit(); } } ] } ] }; if (Ext.is.Phone) { formBase.fullscreen = true; } else { //if desktop Ext.apply(formBase, { autoRender: true, floating: true, modal: true, centered: true, hideOnMaskTap: false, height: 385, width: 480 }); } //create form panel form = new Ext.form.FormPanel(formBase); form.show(); //render the form to the body } });
- Include the following line in
index.html
:<script type="text/javascript" charset="utf-8" src="ch02/ch02_01.js"></script>
- Deploy and access it from the browser. You will see the following screen:
How it works...
The code creates a form panel, with a field set inside it. The field set has four fields specified as part of its child items. xtype
mentioned for each field instructs the Sencha Touch component manager which class to use to instantiate them.
form = new Ext.form.FormPanel(formBase)
creates the form and the other field components using the config defined as part of the formBase.
form.show()
renders the form to the body and that is how it will appear on the screen.
url
contains the URL where the form data will be posted upon submission. The form can be submitted in the following two ways:
form.reset()
resets the status of the form and its fields to the original state. Therefore, if you had entered the values in the fields and clicked on the Reset button, all the fields would be cleared.
form.submit()
posts the form data to the specified url
. The data is posted as an Ajax request using the POST
method.
Use of useClearIcon
on the field instructs Sencha Touch whether it should show the clear icon in the field when the user starts entering a value in it. On clicking on this icon, the value in the field is cleared.
There's more...
In the preceding code, we saw how to construct a form panel, add fields to it, and handle events. We will see what other non-trivial things we may have to do in the project and how we can achieve these using Sencha Touch.
This is the old and traditional way for form data posting to the server url
. If your application need is to use the standard form submit, rather than Ajax, then you will have to set standardSubmit
to true
on the form panel. This is set to false
, by default. The following code snippet shows the usage of this property:
var formBase = { scroll: 'vertical', standardSubmit: true, ...
After this property is set to true
on the FormPanel
, form.submit()
will load the complete page specified in url
.
As we saw earlier, the form data is automatically posted to the url
if the action event (when the Go or Enter key is hit) occurs. In many applications, this default feature may not be desirable. In order to disable this feature, you will have to set submitOnAction
to false
on the form panel.
Say we posted our data to the url
. Now, either the call may fail or it may succeed. In order to handle these specific conditions and act accordingly, we will have to pass additional config options to the form's submit()
method. The following code shows the enhanced version of the submit
call:
form.submit({ success: function(form, result) { Ext.Msg.alert("INFO", "Form submitted!"); }, failure: function(form, result) { Ext.Msg.alert("INFO", "Form submission failed!"); } });
If the Ajax call (to post form data) fails, then the failure
callback function is called, and in the case of success, the success
callback function is called. This works only if the standardSubmit
is set to false
.
See also
- The recipe named Setting up the Android-based development environment in Chapter 1
- The recipe named Setting up the iOS-based development environment in Chapter 1
- The recipe named Setting up the Blackberry-based development environment in Chapter 1
- The recipe named Setting up the browser-based development environment in Chapter 1
- The recipe named Setting up the production environment in Chapter 1