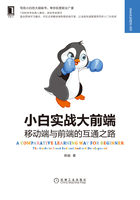
2.2.3 Activity的跳转方法
所有页面都会涉及多个页面之间的跳转,和前端的HTML跳转一样,只不过移动端页面的跳转是Activity之间的跳转,并且Android App的跳转也是可以传递参数的。
下面我们先实现一个从一个Activity跳转到另一个Activity的操作,实现这个操作要4步。
第1步,新建两个Activity。本书前面介绍过,但凡有界面的地方就一定有Activity,况且我们要实现的功能还是一个Activity跳转到另一个Activity,所以第一步要新建两个Activity。在项目的com.example.chenchen.book下创建两个文件,一个是AActivity.java,另一个是BActivity.java,如代码清单2-8、代码清单2-9所示。
代码清单2-8 AActivity代码实现
package com.example.chenchen.book; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; public class AActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_a); } }
代码清单2-9 BActivity代码实现
package com.example.chenchen.book; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; public class BActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_b); } }
代码清单2-8、代码清单2-9就是我们新建一个Android项目时,默认创建的Activity的代码。大家看到代码清单2-8和代码清单2-9中都有一行代码执行了setContentView()的函数,setContentView()函数就是我们之前提过的设置App的界面文件。
第2步,我们需要编写两个xml文件,作为AActivity和BActivity的界面。AActivity对应的界面文件名字叫activity_a.xml,具体的代码实现见代码清单2-10。
代码清单2-10 activity_a.xml代码实现
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="我是AActivity" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" /> <Button android:layout_width="150dp" android:layout_height="30dp" android:text="跳转到B" android:id="@+id/toB" /> </android.support.constraint.ConstraintLayout>
activity_a.xml中的部分代码之前配置Activity时介绍过。代码清单2-10中需要关注的是<Button>标签,因为当我们从AActivity跳转到BActivity的时候需要点击这个按钮触发跳转事件,另外在<Button>标签中出现了之前没有见过的属性,就是id。这个属性声明了<Button>标签的id为toB,后续我们在Activity中编写逻辑代码的时候,可以通过查找界面的xml中id为toB的标签来获取这个<Button>标签。(id在绑定事件的时候比较常用。)
相比之下,activity_b.xml的代码就简单一点,就只有一个<TextView>标签,用于标记展示的Activity是AActivity还是BActivity,具体如代码清单2-11所示。
代码清单2-11 activity_b.xml代码实现
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="我是BActivity" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" /> </android.support.constraint.ConstraintLayout>
第3步,为AActivity界面中的按钮添加点击事件,具体如代码清单2-12所示。在代码清单2-12中,先找到要绑定事件的标签,即之前设置id属性的那个按钮。在Android编程过程中,大多数xml界面文件中的标签获取都是通过findViewByIdid函数来进行获取,然后把对应属性的id当作参数传入到函数中就可以了。
之后,通过setOnClickListener函数绑定这个按钮的点击事件,并在点击事件里加入跳转的功能代码。我们通过创建Intent对象的方式来实现,Intent初始化的时候会传入两个参数,第1个参数为当前AActivity的上下文,也就是AActivity.this属性,第2个参数是目标Activity的class属性,也就是BActivity.class。启动跳转的操作通过AActivity.this.startActivity()函数实现,把我们刚刚初始化的Intent传入AActivity.this.startActivity()函数中,就能实现界面从AActivity跳转到BActivity。
代码清单2-12 AActivity.java代码实现
package com.example.chenchen.book; import android.content.Intent; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.Button; public class AActivity extends AppCompatActivity { AActivity mContext = this; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_a); Button button = findViewById(R.id.toB); button.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent intent = new Intent(AActivity.this,BActivity.class); mContext.startActivity(intent); } }); } }
但是在这个时候,并不代表AActivity跳转BActivity这个功能就做完了,因为我们还没有更改启动时的Activity配置。该配置在2.2.2节提到的文件AndroidMani-fest.xml中,所以第4步需要把intent-filter标签以及里面的嵌套标签用AActivity的<Activity>标签包裹起来,具体修改代码如代码清单2-13所示。
代码清单2-13 修改App启动入口为AActivity
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.chenchen.book"> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> </activity> <activity android:name=".AActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name=".BActivity"> </activity> </application> </manifest>}
当我们把上面这些配置文件配置完成之后,在启动App时就默认会启动AActivity,启动之后的界面如图2-10所示。

图2-10 App启动时的AActivity界面
在图2-10界面中点击“跳转到B”选项后,我们就会看到图2-11中的界面。到这里,Activity之间的基础跳转就完成了。

图2-11 AActivity跳转到BActivity之后的界面