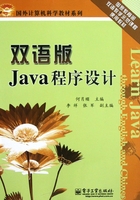
4.3 Boolean Logical Operators
The boolean logical operators shown here operate only on boolean operands. All of the binary logical operators combine two boolean values to form a resultant boolean value. Table 4.3 lists logical operators.
Table 4.3 Logical Operators
class example4 { public static void main(String args[]) { boolean b; b = (2 > 3) && (3 < 2); System.out.println("b = "+b); b = false || true ; System.out.println("b = "+b); } }
The output of the program is shown here:
b = false
b = true
Ternary Operator
Java includes a special ternary (three-way) operator that can replace certain types of if-then-else statement.
Java包含一个特殊的运算符(三元运算符),可以替代语句if-then-else类型。
expression1 ? expression2 : expression3
Here, expression1 can be any expression that evaluates to a boolean value. If expression1 is true, then expression2 is evaluated; otherwise, expression3 is evaluated. The result of the ? operation is that of the expression evaluated. Both expression2 and expression3 are required to return the same type, which can’t be void.
先求解表达式1的布尔值,若为真则求解表达式2的值,否则求解表达式3的值。?运算的结果是被求解的表达式的值。要求表达式2和表达式3返回相同类型的值,这个值不能为空。
eg: int i=20; int j=30; int max = ( i > j ) ? x : j;
this section checks for the condition, if i is greater than j then value of i is assigned to max else value of j is assigned to max.
class example5 { public static void main(String args[]) { int i,k; i = 10; k = i < 0 ? -i : i; System.out.print("Absolute value of "); System.out.println(i + " is " + k); i = -10; k = i < 0 ? -i : i; //get absolute value of i System.out.print("Absolute value of "); System.out.println(i + " is " + k); } }
when you run this program you will get following output:
Absolute value of 10 is 10
Absolute value of -10 is 10