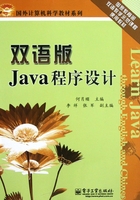
4.4 Bitwise and Shift Operators
The bitwise and shift operators are low-level operators that manipulate the individual bits that make up an integer value. The bitwise operators are most commonly used for testing and setting individual flag bits in a value. In order to understand their behavior, you must understand binary (base-2) numbers and the twos-complement format used to represent negative integers. You cannot use these operators with floating-point, boolean, array, or object operands. When used with boolean operands, the &, |, and ^ operators perform a different operation, as described in the previous section.
If either of the arguments to a bitwise operator is a long, the result is a long. Otherwise, the result is an int. If the left operand of a shift operator is a long, the result is a long; otherwise, the result is an int.
位运算和移位运算是底层的运算,可以实现将单个的二进制位组装成整型。位运算符通常被用来测试和设置单个二进制位的值。如果位运算操作数是long型,其结果也是long型;否则,结果就是int型。
4.4.1 Bitwise Complement (~)
The unary ~ operator is known as the bitwise complement, or bitwise NOT, operator. It inverts each bit of its single operand, converting ones to zeros and zeros to ones. For example:
byte b = ~12; // ~00000110 ==> 11111001 or -13 decimal flags = flags & ~f;
4.4.2 Bitwise AND (&)
This operator combines its two integer operands by performing a Boolean AND operation on their individual bits. The result has a bit set only if the corresponding bit is set in both operands. For example:
&运算符将参加运算的两个整数操作数按二进制位进行布尔AND运算。如果相应的二进制位都为1,则该位的结果为1。
10 & 7 // 00001010 & 00000111 ==> 00000010 or 2 if ((flags & f) != 0)
When used with boolean operands, & is the infrequently used Boolean AND operator described earlier.
4.4.3 Bitwise OR ( | )
This operator combines its two integer operands by performing a Boolean OR operation on their individual bits. The result has a bit set if the corresponding bit is set in either or both of the operands. It has a zero bit only where both corresponding operand bits are zero. For example:
|运算符将参加运算的两个整数操作数按二进制位进行布尔OR运算。如果相应的二进制位为1或者全都为1,则该位的结果为1。相应的二进制位全都为0,则该位的结果为0。
10 | 7 // 00001010 | 00000111 ==> 00001111 or 15 flags = flags | f;
When used with boolean operands, | is the infrequently used Boolean OR operator described earlier.
4.4.4 Bitwise XOR (^)
This operator combines its two integer operands by performing a Boolean XOR (Exclusive OR) operation on their individual bits. The result has a bit set if the corresponding bits in the two operands are different. If the corresponding operand bits are both ones or both zeros, the result bit is a zero. For example:
10 & 7 // 00001010 ^ 00000111 ==> 00001101 or 13
When used with boolean operands, ^ is the infrequently used Boolean XOR operator.
4.4.5 Left Shift (<<)
The << operator shifts the bits of the left operand left by the number of places specified by the right operand. High-order bits of the left operand are lost, and zero bits are shifted in from the right. Shifting an integer left by n places is equivalent to multiplying that number by 2n. For example:
<<运算符将它左边的操作数的二进制数左移该运算符右边操作数指定的位数。
10 << 1 // 00001010 << 1 = 00010100 = 20 = 10*2 7 << 3 // 00000111 << 3 = 00111000 = 56 = 7*8 -1 << 2 // 0xFFFFFFFF << 2 = 0xFFFFFFFC = -4 = -1*4
If the left operand is a long, the right operand should be between 0 and 63. Otherwise, the left operand is taken to be an int, and the right operand should be between 0 and 31.
4.4.6 Signed Right Shift (>>)
The >> operator shifts the bits of the left operand to the right by the number of places specified by the right operand. The low-order bits of the left operand are shifted away and are lost. The high-order bits shifted in are the same as the original high-order bit of the left operand. In other words, if the left operand is positive, zeros are shifted into the high-order bits. If the left operand is negative, ones are shifted in instead. This technique is known as sign extension; it is used to preserve the sign of the left operand. For example:
>>运算符将它左边的操作数的二进制数右移该运算符右边操作数指定的位数。如果左边的这个操作数是正数,移位后高位补0。如果左边的这个操作数是负数,移位后高位补1。这被称为符号扩展,常用来保存左边操作数的符号。
10 >> 1 // 00001010 >> 1 = 00000101 = 5 = 10/2 27 >> 3 // 00011011 >> 3 = 00000011 = 3 = 27/8 -50 >> 2 // 11001110 >> 2 = 11110011 = -13 != -50/4
If the left operand is positive and the right operand is n, the >> operator is the same as integer division by 2n.
4.4.7 Unsigned Right Shift (>>>)
This operator is like the >> operator, except that it always shifts zeros into the high-order bits of the result, regardless of the sign of the left-hand operand. This technique is called zero extension; it is appropriate when the left operand is being treated as an unsigned value (despite the fact that Java integer types are all signed). Examples:
-50 >>> 2 // 11001110 >>> 2 = 00110011 = 51 0xff >>> 4 // 11111111 >>> 4 = 00001111 = 15 = 255/16