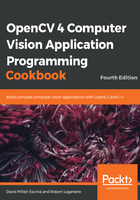
上QQ阅读APP看书,第一时间看更新
How to do it...
The conversion of images between different color spaces is easily done through the use of the cv::cvtColor OpenCV function, as follows:
- Let's convert the input image to the CIE L*a*b* color space at the beginning of the process method:
cv::Mat ColorDetector::process(const cv::Mat &image) { // re-allocate binary map if necessary // same size as input image, but 1-channel result.create(image.rows,image.cols,CV_8U); // Converting to Lab color space cv::cvtColor(image, converted, cv::COLOR_BGR2Lab); // get the iterators of the converted image cv::Mat_<cv::Vec3b>::iterator it= converted.begin<cv::Vec3b>(); cv::Mat_<cv::Vec3b>::iterator itend= converted.end<cv::Vec3b>(); // get the iterator of the output image cv::Mat_<uchar>::iterator itout= result.begin<uchar>(); // for each pixel for ( ; it!= itend; ++it, ++itout) { ...
- The converted variable contains the image after color conversion. In the ColorDetector class, it is defined as a class attribute:
class ColorDetector { private: // image containing color converted image cv::Mat converted;
- You also need to convert the input target color. You can do this by creating a temporary image that contains only one pixel. Note that you need to keep the same signature as in the earlier recipes; that is, the user continues to supply the target color in RGB:
// Sets the color to be detected void setTargetColor(unsigned char red, unsigned char green, unsigned char blue) { // Temporary 1-pixel image cv::Mat tmp(1,1,CV_8UC3); tmp.at<cv::Vec3b>(0,0)= cv::Vec3b(blue, green, red); // Converting the target to Lab color space cv::cvtColor(tmp, tmp, cv::COLOR_BGR2Lab); target= tmp.at<cv::Vec3b>(0,0); }
If the application of the preceding recipe is compiled with this modified class, it will now detect the pixels of the target color using the CIE L*a*b* color model.