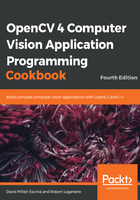
How it works...
When an image is converted from one color space to another, a linear or nonlinear transformation is applied on each input pixel to produce the output pixels. The pixel type of the output image will match one of the input images. Even if you work with 8-bit pixels most of the time, you can also use a color conversion with floating-point images (in which case, the pixel values are generally assumed to vary between 0 and 1.0) or with integer images (with pixels generally varying between 0 and 65535). However, the exact domain of the pixel values depends on the specific color space and destination image type. For example, with the CIE L*a*b* color space, the L channel, which represents the brightness of each pixel, varies between 0 and 100, and it is rescaled between 0 and 255 in the cases of 8-bit images. The a and b channels correspond to the chromaticity components. These channels contain information about the color of a pixel, independent of its brightness. Their values vary between -127 and 127; for 8-bit images, 128 is added to each value in order to make it fit within the 0 to 255 interval. However, note that the 8-bit color conversion will introduce rounding errors that will make the transformation imperfectly reversible.
Most commonly used color spaces are available. It is just a question of providing the right color space conversion code to the OpenCV function (for CIE L*a*b*, this code is cv::COLOR_BGR2Lab). Among these is YCrCb, which is the color space used in JPEG compression. To convert a color space from BGR to YCrCb, the code will be cv::COLOR_BGR2YCrCb. Note that all the conversions that involve the three regular primary colors, red, green, and blue, are available in the RGB and BGR order.
The CIE L*u*v* color space is another perceptually uniform color space. You can convert from BGR to CIE L*u*v by using the cv::COLOR_BGR2Luv code. Both L*a*b* and L*u*v* use the same conversion formula for the brightness channel, but use a different representation for the chromaticity channels. Also, note that since these two color spaces distort the RGB color domain in order to make it perceptually uniform, these transformations are nonlinear (therefore, they are costly to compute).
There is also the CIE XYZ color space (converted using the cv::COLOR_BGR2XYV code). It is a standard color space used to represent any perceptible color in a device-independent way. This space is not usually directly used while processing. In the computation of the L*u*v and L*a*b color spaces, the XYZ color space is used as an intermediate representation. The transformation between RGB and XYZ is linear. It is also interesting to note that the Y channel corresponds to a gray-level version of the image.
HSV and HLS are interesting color spaces because they decompose the colors into their hue and saturation components, plus their value or luminance component, which is a more natural way for humans to describe colors.
You can also convert color images to a gray-level intensity. The output will be a one-channel image:
cv::cvtColor(color, gray, cv::COLOR_BGR2Gray);
It is also possible to make conversions in another direction, but the three channels of the resulting color image will then be filled identically with the corresponding values in the gray-level image.