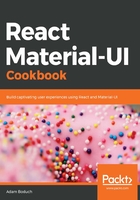
上QQ阅读APP看书,第一时间看更新
How to do it...
Let's say that you want to support different types of drawers in your app. You can control the Drawer component type using the variant property. Here's the code:
import React, { useState } from 'react';
import Drawer from '@material-ui/core/Drawer';
import Grid from '@material-ui/core/Grid';
import Button from '@material-ui/core/Button';
import List from '@material-ui/core/List';
import ListItem from '@material-ui/core/ListItem';
import ListItemIcon from '@material-ui/core/ListItemIcon';
import ListItemText from '@material-ui/core/ListItemText';
export default function DrawerTypes({ classes, variant }) {
const [open, setOpen] = useState(false);
return (
<Grid container justify="space-between">
<Grid item>
<Drawer
variant={variant}
open={open}
onClose={() => setOpen(false)}
>
<List>
<ListItem
button
onClick={() => setOpen(false)}
>
<ListItemText>Home</ListItemText>
</ListItem>
<ListItem
button
onClick={() => setOpen(false)}
>
<ListItemText>Page 2</ListItemText>
</ListItem>
<ListItem
button
onClick={() => setOpen(false)}
>
<ListItemText>Page 3</ListItemText>
</ListItem>
</List>
</Drawer>
</Grid>
<Grid item>
<Button onClick={() => setOpen(!open)}>
{open ? 'Hide' : 'Show'} Drawer
</Button>
</Grid>
</Grid>
);
}
The variant property defaults to temporary. When you first load this screen, you'll only see the button to toggle the drawer display:

When you click on this button, you'll see a temporary drawer:
