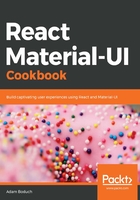
How it works...
Before you start changing the variant property, let's walk through the code in this example, starting with the Drawer markup, as follows:
<Drawer
variant={variant}
open={open}
onClose={() => setOpen(false)}
>
<List>
<ListItem
button
onClick={() => setOpen(false)}
>
<ListItemText>Home</ListItemText>
</ListItem>
<ListItem
button
onClick={() => setOpen(false)}
>
<ListItemText>Page 2</ListItemText>
</ListItem>
<ListItem
button
onClick={() => setOpen(false)}
>
<ListItemText>Page 3</ListItemText>
</ListItem>
</List>
</Drawer>
The Drawer component takes an open property, which displays the drawer when true. The variant property determines the type of drawer to render. The screenshot shown previously is a temporary drawer, the default variant value. The Drawer component has List as its child, where each of the items displayed in the drawer are rendered.
Next, let's take a look at the Button component that toggles the display of the Drawer component:
<Button onClick={() => setOpen(!open)}>
{open ? 'Hide' : 'Show'} Drawer
</Button>
When this button is clicked, the open state of your component is toggled. Likewise, the text of the button is toggled depending on the value of the open state.
Now let's try changing the value of the variant property to permanent. Here's what the drawer looks like when rendered:

A permanent drawer, as the name suggests, is always visible and is always in the same place on the screen. If you click on the SHOW DRAWER button, the open state of your component is toggled to true. You'll see the text of the button change, but since the Drawer component is using the permanent variant, the open property has no effect:

Next, let's try the persistent variant. Persistent drawers are similar to permanent drawers in that they stay visible on the screen while the user interacts with the app, and they're similar to temporary drawers in that they can be hidden by changing the open property.
Let's change the variant property to persistent. When the screen first loads, the drawer isn't visible because the open state of your component is false. Try clicking on the SHOW DRAWER button. The drawer is displayed, and it looks like the permanent drawer. If you click the HIDE DRAWER button, the open state of your component is toggled to false and the drawer is hidden.
Persistent drawers should be used when you want the user to be able to control the visibility of the drawer. For example, with temporary drawers the user can close the drawer by clicking on the overlay or by hitting the Esc key. Permanent drawers are useful when you want to use the left-hand navigation as an integral part of the page layout—they are always visible and other items are laid out around them.