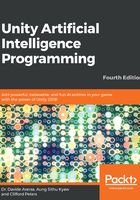
上QQ阅读APP看书,第一时间看更新
The abstract FSM class
Next, we implement a generic abstract class to define the methods that our enemy tank AI class has to implement.
The code in the FSM.cs file is as follows:
using UnityEngine; using System.Collections; public class FSM : MonoBehaviour { //Player Transform protected Transform playerTransform; //Next destination position of the NPC Tank protected Vector3 destPos; //List of points for patrolling protected GameObject[] pointList; //Bullet shooting rate protected float shootRate; protected float elapsedTime; //Tank Turret public Transform turret { get; set; } public Transform bulletSpawnPoint { get; set; } protected virtual void Initialize() { } protected virtual void FSMUpdate() { } protected virtual void FSMFixedUpdate() { } // Use this for initialization void Start () { Initialize(); } // Update is called once per frame void Update () { FSMUpdate(); } void FixedUpdate() { FSMFixedUpdate(); } }
The enemy tanks need only to know the position of the player's tank, their next destination point, and the list of waypoints to choose from while they're patrolling. Once the player tank is in range, they rotate their turret object and then start shooting from the bullet spawn point at their fire rate.
The inherited classes will also need to implement the three methods: Initialize, FSMUpdate, and FSMFixedUpdate.