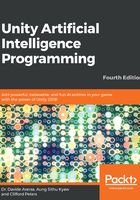
The enemy tank AI
Let's look at the real code for our AI tanks. Let's create a new class, called SimpleFSM, which inherits from our FSM abstract class.
The code in the SimpleFSM.cs file is as follows:
using UnityEngine; using System.Collections; public class SimpleFSM : FSM { public enum FSMState { None, Patrol, Chase, Attack, Dead, } //Current state that the NPC is reaching public FSMState curState; //Speed of the tank private float curSpeed; //Tank Rotation Speed private float curRotSpeed; //Bullet
[SerializeField] private GameObject Bullet; //Whether the NPC is destroyed or not private bool bDead; private int health;
// We overwrite the deprecated built-in `rigidbody` variable.
new private Rigidbody rigidbody;
Here, we declare a few variables. Our tank AI will have four different states: Patrol, Chase, Attack, and Dead. Basically, we are implementing the FSM that we described as an example in Chapter 1, Introduction to AI:
In our Initialize method, we set up our AI tank's properties with default values. Then we store the positions of waypoints in our local variable. We got those waypoints from our scene using the FindGameObjectsWithTag method, trying to find those objects with the WandarPoint tag:
//Initialize the Finite state machine for the NPC tank protected override void Initialize () { curState = FSMState.Patrol; curSpeed = 150.0f; curRotSpeed = 2.0f; bDead = false; elapsedTime = 0.0f; shootRate = 3.0f; health = 100; //Get the list of points pointList = GameObject.FindGameObjectsWithTag("WandarPoint"); //Set Random destination point first FindNextPoint(); //Get the target enemy(Player) GameObject objPlayer = GameObject.FindGameObjectWithTag("Player");
// Get the rigidbody
rigidbody = GetComponent<Rigidbody>(); playerTransform = objPlayer.transform; if (!playerTransform) print("Player doesn't exist.. Please add one "+ "with Tag named 'Player'"); //Get the turret of the tank turret = gameObject.transform.GetChild(0).transform; bulletSpawnPoint = turret.GetChild(0).transform; }
The Update method that gets called every frame looks as follows:
//Update each frame protected override void FSMUpdate() { switch (curState) { case FSMState.Patrol: UpdatePatrolState(); break; case FSMState.Chase: UpdateChaseState(); break; case FSMState.Attack: UpdateAttackState(); break; case FSMState.Dead: UpdateDeadState(); break; } //Update the time elapsedTime += Time.deltaTime; //Go to dead state is no health left if (health <= 0) curState = FSMState.Dead; }
We check the current state and then call the appropriate state method. Once the health object has a value of zero or less, we set the tank to the Dead state.