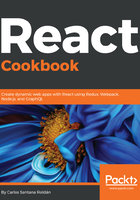
上QQ阅读APP看书,第一时间看更新
How to do it...
We will now go about adding CSS to our Home component:
- Create a new application, or use the previous one (my-first-react-app).
- Then create a new CSS file for our Home component. Let's reuse the Home component we created in the last recipe. Now you need to create a Home.css file at the same level as your Home.js file (inside the components folder). Before you create this file, let's modify our Home component a little bit:
import React, { Component } from 'react';
// We import our Home.css file here
import './Home.css';
class Home extends Component {
render() {
return (
<div className="Home">
<h1>Welcome to Codejobs</h1>
<p>
In this recipe you will learn how to add styles to
components. If you want to learn more you can visit
our Youtube Channel at
<a href="http://youtube.com/codejobs">Codejobs</a>.
</p>
</div>
);
}
}
export default Home;
File: src/components/Home/Home.js
- We'll now add styles to our Home.css. Basically, we wrapped our component into a div with a className of Home, and inside we have an <h1> tag with the text Welcome to Codejobs, and then a <p> tag with a message. We need to import our Home.css file directly, and then our CSS file will look like this:
.Home {
margin: 0 auto;
width: 960px;
}
.Home h1 {
font-size: 32px;
color: #333;
}
.Home p {
color: #333;
text-align: center;
}
.Home a {
color: #56D5FA;
text-decoration: none;
}
.Home a:hover {
color: #333;
}
File: src/components/Home/Home.css
- Now let's suppose you need to add an inline style. We do this with the style property, and the CSS properties need to be written in camelCase and between {{ }}, like this:
import React, { Component } from 'react';
// We import our Home.css file here
import './Home.css';
class Home extends Component {
render() {
return (
<div className="Home">
<h1>Welcome to Codejobs</h1>
<p>
In this recipe you will learn how to add styles to
components. If you want to learn more you can visit
our Youtube Channel at
<a href="http://youtube.com/codejobs">Codejobs</a>.
</p>
<p>
<button
style={{
backgroundColor: 'gray',
border: '1px solid black'
}}
>
Click me!
</button>
</p>
</div>
);
}
}
export default Home;
File: src/components/Home/Home.js
- You also can pass an object to the style property like this:
import React, { Component } from 'react';
// We import our Home.css file here
import './Home.css';
class Home extends Component {
render() {
// Style object...
const buttonStyle = {
backgroundColor: 'gray',
border: '1px solid black'
};
return (
<div className="Home">
<h1>Welcome to Codejobs</h1>
<p>
In this recipe you will learn how to add styles to
components. If you want to learn more you can visit
our Youtube Channel at
<a href="http://youtube.com/codejobs">Codejobs</a>.
</p>
<p>
<button style={buttonStyle}>Click me!</button>
</p>
</div>
);
}
}
export default Home;
File: src/components/Home/Home.js