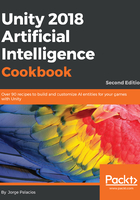
上QQ阅读APP看书,第一时间看更新
How to do it...
Pursue and Evade are essentially the same algorithm, but differ in terms of the base class they derive from:
- Create the Pursue class, derived from Seek, and add the attributes for the prediction:
using UnityEngine; using System.Collections; public class Pursue : Seek { public float maxPrediction; private GameObject targetAux; private Agent targetAgent; }
- Implement the Awake function in order to set up everything according to the real target:
public override void Awake() { base.Awake(); targetAgent = target.GetComponent<Agent>(); targetAux = target; target = new GameObject(); }
- Implement the OnDestroy function for properly handling the internal object:
void OnDestroy () { Destroy(targetAux); }
- Implement the GetSteering function:
public override Steering GetSteering() { Vector3 direction = targetAux.transform.position - transform.position; float distance = direction.magnitude; float speed = agent.velocity.magnitude; float prediction; if (speed <= distance / maxPrediction) prediction = maxPrediction; else prediction = distance / speed; target.transform.position = targetAux.transform.position; target.transform.position += targetAgent.velocity * prediction; return base.GetSteering(); }
- To create the Evade behavior, the procedure is just the same, but it takes into account the fact that Flee is the parent class:
public class Evade : Flee { // everything stays the same }