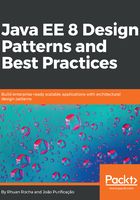
Implementing the EmployeeStoreManager class
The EmployeeStoreManager class is responsible for connecting with the data source as well as reading and writing employee data:
import com.packt.javaee8.entity.Employee;
import java.util.HashMap;
import java.util.Map;
public class EmployeeStoreManager {
private Map<Object, Employee> dataSource = new HashMap<>();
public void storeNew ( Employee employee ) throws Exception {
if( dataSource.containsKey( employee.getId() ) ) throw new Exception( "Data already exist" );
dataSource.put( employee.getId(), employee );
}
public void update ( Employee employee ) throws Exception {
if( !dataSource.containsKey( employee.getId() ) ) throw new Exception( "Data not exist" );
dataSource.put( employee.getId(), employee );
}
public void delete ( Employee employee ) throws Exception {
if( !dataSource.containsKey( employee.getId() ) ) throw new Exception( "Data not exist" );
dataSource.remove( employee.getId() );
}
public Employee load(Object key){
return dataSource.get( key );
}
}
In the preceding code block, we have the EmployeeStoreManager class, which is responsible for connecting with the data source and reading and writing employee data. This class works as a DAO and encapsulates all the data source complexity. It also has the dataSource attribute, which is a map that represents a data source. Furthermore, this class has the storeNew method, which is used to write new employee data represented by the object model. It also has the update method, used to write existing employee data represented by an object model. This method is used to update stored data. The delete method is also used to delete existing employee data, and the load method is used to read employee data in an object model.