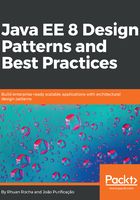
Implementing the StageManager interface
StageManager is an interface that is implemented by EmployeeStageManager:
public interface StageManager {
public void flush();
public void load();
public Entity getEntity();
}
In the preceding code block, we have the StageManager interface. This interface is implemented by EmployeeStageManager:
public class EmployeeStageManager implements StageManager {
private boolean isNew;
private Employee employee;
public EmployeeStageManager ( Employee employee ){
this.employee = employee;
}
public void flush(){
EmployeeStoreManager employeeStoreManager = new EmployeeStoreManager();
if( isNew ){
try {
employeeStoreManager.storeNew( employee );
} catch ( Exception e ) {
e.printStackTrace();
}
isNew = false;
}
else {
try {
employeeStoreManager.update( employee );
} catch ( Exception e ) {
e.printStackTrace();
}
}
}
public void load() {
EmployeeStoreManager storeManager =
new EmployeeStoreManager();
Employee empl = storeManager.load( employee.getId() );
updateEmployee( empl );
}
private void updateEmployee( Employee empl ) {
employee.setId( empl.getId() );
employee.setAddress( empl.getAddress() );
employee.setName( empl.getName() );
employee.setSalary( empl.getSalary() );
isNew = false;
}
public Entity getEntity() {
return employee;
}
}
In the preceding code block, we have the isNew attribute, which is used to define whether or not the object model is a new write (new data will be created on data source). The code also contains the employee attribute, which is the object model used to represent employee data. We can also see the flush method, used to execute the process for writing data on the data source; the load method, used to read data from the data source; the updateEmployee method, which is the private method used to update the employee attribute; and the getEntity method, which is used to return the employee attribute.