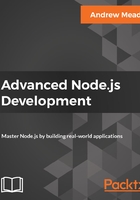
Using object destructuring ES6
Object destructuring lets you pull out properties from an object in order to create variables. This means that if we have an object called user and it's set equal to an object with a name property set to andrew and an age property set to 25, as shown in the following code:
const MongoClient = require('mongodb').MongoClient;
var user = {name: 'andrew', age: 25};
We can easily pull out one of these into a variable. Let's say, for example, we want to grab name and create a name variable. To do that using object destructuring in ES6, we're going to make a variable and then we're going to wrap it inside of curly braces. We're going to provide the name we want to pull out; this is also going to be the variable name. Then, we're going to set it equal to whatever object we want to destructure. In this case, that is the user object:
var user = {name: 'andrew', age: 25};
var {name} = user;
We have successfully destructured the user object, pulling off the name property, creating a new name variable, and setting it equal to whatever the value is. This means I can use the console.log statement to print name to the screen:
var user = {name: 'andrew', age: 25};
var {name} = user;
console.log(name);
I'm going to rerun the script and we get andrew, which is exactly what you'd expect because that is the value of the name property:

ES6 destructuring is a fantastic way to make new variables from an object's properties. I'm going to go ahead and delete this example, and at the top of the code, we're going to change our require statement so that it uses destructuring.
Before we add anything new, let's go ahead and take the MongoClient statement and switch it to destructuring; then, we'll worry about grabbing that new thing that's going to let us make ObjectIds. I'm going to copy and paste the line and comment out the old one so we have it for reference.
// const MongoClient = require('mongodb').MongoClient;
const MongoClient = require('mongodb').MongoClient;
What we're going to do is remove our .MongoClient call after require. There's no need to pull off that attribute because we're going to be using destructuring instead. That means over here we can use destructuring, which requires us to add our curly braces, and we can pull off any property from the MongoDB library.
const {MongoClient} = require('mongodb');
In this case, the only property we had was MongoClient. This creates a variable called MongoClient, setting it equal to the MongoClient property of require('mongodb'), which is exactly what we did in the previous require statement.