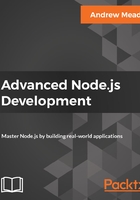
Creating a new instance of objectID
Now that we have some destructuring in place, we can easily pull more things off of MongoDB. We can add a comma and specify something else we want to pull off. In this case, we're going to pull off uppercase, ObjectID.
const {MongoClient, ObjectID} = require('mongodb');
This ObjectID constructor function lets us make new ObjectIds on the fly. We can do anything we like with them. Even if we're not using MongoDB as our database, there is some value in creating and using ObjectIds to uniquely identify things. Next, we can make a new ObjectId by first creating a variable. I'll call it obj, and we'll set it equal to new ObjectID, calling it as a function:
const {MongoClient, ObjectID} = require('mongodb');
var obj = new ObjectID();
Using the new keyword, we can create a new instance of ObjectID. Next up, we can go ahead and log that to the screen using console.log(obj). This is a regular ObjectId:
console.log(obj);
If we rerun the file over from the Terminal, we get exactly what you'd expect:

We get an ObjectId-looking thing. If I rerun it again, we get a new one; they are both unique:

Using this technique, we can incorporate ObjectIds anywhere we like. We could even generate our own, setting them as the _id property for our documents, although I find it much easier to let MongoDB handle that heavy lifting for us. I'm going to go ahead and remove the following two lines since we won't actually be using this code in the script:
var obj = new ObjectID(); console.log(obj);
We have learned a bit about ObjectIds, what they are, and why they're useful. In the following sections, we're going to be taking a look at other ways we can work with MongoDB. We'll learn how to read, remove, and update our documents.