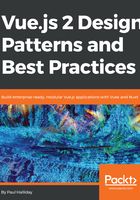
Loading modules without Webpack
Although Webpack helps us in more ways than simply loading a module, we can load a JavaScript module at this moment in time natively in the browser. It tends to perform worse than bundling tools (at the time of writing), but this may change over time.
To demonstrate this, let's head over to the terminal and make a simple counter with a project based on the simple template. This template effectively starts a new Vue project without any bundling tools:
# New Vue Project
vue init simple vue-modules
# Navigate to Directory
cd vue-modules
# Create App and Counter file
touch app.js
touch counter.js
We can then edit our index.html to add script files from type="module" this allows us to use the export/import syntax outlined before:
<!-- index.html -->
<!DOCTYPE html>
<html>
<head>
<title>Vue.js Modules - Counter</title>
<script src="https://unpkg.com/vue"></script>
</head>
<body>
<div id="app">
</div>
<script type="module" src="counter.js"></script>
<script type="module" src="app/app.js"></script>
</body>
</html>
Warning: Ensure that your browser is up to date so that the preceding code can run successfully.
Then, inside of our counter.js, we can export a new default object, which acts as a new Vue instance. It acts as a simple counter that allows us to either increment or decrements a value:
export default {
template: `
<div>
<h1>Counter: {{counter}}</h1>
<button @click="increment">Increment</button>
<button @click="decrement">Decrement</button>
</div>`,
data() {
return {
counter: 1
};
},
methods: {
increment() {
this.counter++;
},
decrement() {
this.counter--;
}
}
};
We can then import the counter.js file inside of app.js, thus demonstrating the ways we can import/export modules. To get our counter to display inside of our root Vue instance, we're registering the counter as a component inside this instance, and setting the template to <counter></counter>, the name of our component:
import Counter from './counter.js';
const app = new Vue({
el: '#app',
components: {
Counter
},
template: `<counter></counter>`
});
We'll look at this in more detail in future sections of the book, but all you need to know at this point is that it effectively acts as another Vue instance. When we register the component inside of our instance, we're only able to access this component from that instance.
Awesome! Here are the results of our module import/exports:

In the next section, we'll take a deeper look at debugging our Vue applications, and the role Vue devtools plays in this.