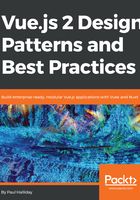
Integrating with Vue
To integrate RxJS with Vue, we'll need to make a new Vue project and install both RxJS and Vue-Rx. One of the great things about using the Vue-Rx plugin is that it's officially supported by the Vue.js team, which gives us confidence that it'll be supported in the long term.
Let's create a new project with the Vue CLI, and integrate RxJS:
# New Vue project
vue init webpack-simple vue-rxjs
# Change directory
cd vue-rxjs
# Install dependencies
npm install
# Install rxjs and vue-rx
npm install rxjs vue-rx
# Run project
npm run dev
We now need to tell Vue that we'd like to use the VueRx plugin. This can be done using Vue.use(), and is not specific to this implementation. Any time we're looking to add new plugins to our Vue instance(s), calling Vue.use() makes an internal call to the plugin's install() method, extending the global scope with the new functionality. The file to edit will be our main.js file, which is located at src/main.js. We'll be looking at plugins in more detail within later chapters of this book:
import Vue from "vue";
import App from "./App.vue";
import VueRx from "vue-rx";
import Rx from "rxjs";
// Use the VueRx plugin with the entire RxJS library
Vue.use(VueRx, Rx);
new Vue({
el: "#app",
render: h => h(App)
});
Notice any issues with the preceding implementation? Well, in the interests of application performance and reducing bundle size, we should only import what we need. This then becomes:
import Vue from "vue";
import App from "./App.vue";
import VueRx from "vue-rx";
// Import only the necessary modules
import { Observable } from "rxjs/Observable";
import { Subject } from "rxjs/Subject";
// Use only Observable and Subject. Add more if needed.
Vue.use(VueRx, {
Observable,
Subject
});
new Vue({
el: "#app",
render: h => h(App)
});
We can then create an Observable data stream inside of our Vue application. Let's head over to App.vue, and import the necessary modules from RxJS:
// Required to create an Observable stream
import { Observable } from 'rxjs/Observable';
import 'rxjs/add/observable/of';
We can then create a new Observable of data; in this example, we'll be using a simple array of people:
// New Observable stream of string array values
const people$ = Observable.of(['Paul', 'Katie', 'Bob']);
This then allows us to subscribe to this Observable from within the subscriptions object. If you've ever used Angular before, this allows us to access the Observable (and handles the necessary unsubscription) similar to the Async pipe:
export default {
data () {
return {
msg: 'Welcome to Your Vue.js App'
}
},
/**
* Bind to Observable using the subscriptions object.
* Allows us to then access the values of people$ inside of our template.
* Similar to the Async pipe within Angular
**/
subscriptions: {
people$
}
}
As well as this, if we wanted to create a new instance of our Observable for each component, we can instead declare our subscriptions as a function:
subscriptions() {
const people$ = Observable.of(['Paul', 'Katie', 'Bob'])
return {
people$
}
}
Finally, we can then display the results of the Observable inside of our template. We can use the v-for directive to iterate over the array and display the results on screen. How does this work? Using the following example, the v-for syntax uses an item in items syntax, which can be thought of a person in people$ in our context. This allows us to access each item inside of our people$ Observable (or any other array) with interpolation binding:
<template>
<div id="app">
<ul>
<li
v-for="(person,index) in people$":key="index"> {{person}}
</li>
</ul>
</div>
</template>
As you can see inside of the browser, our three items have now appeared on the screen inside of our list item:
