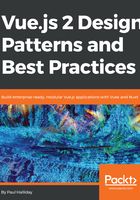
Summary
In this chapter, we looked at how we can take advantage of the Vue CLI to scaffold new Vue projects with appropriate bundling configurations and ES2015 support. We've seen that not only does this give us extra power, but it also saves us a significant amount of time in the long run. We don't have to remember how to create a Webpack or Babel configuration, as this is all handled for us by the starter templates; but even still, if we want to add extra configuration options, we can.
We then looked at how we can implement TypeScript with Webpack and the ts-loader, as well as taking advantage of common TypeScript and Vue patterns with the property decorator(s). This allows us to take advantage of core tooling and help reduce bugs in our code.
Finally, we also implemented RxJS and Vue-Rx in our application to take advantage of the Observable pattern. If you're interested in using RxJS inside of your projects, this is a good starting point for future integrations.
In the next chapter, we're going to take a deeper look at the Vue.js instance and the different reserved properties, such as data, computed, ND watch, as well as creating getters and setters. This chapter especially considers when you should use computed to use or watch properties.
In this section, we'll be investigating how a Vue.js instance works at a lower level by looking at how this is handled by Vue. We'll also be looking at the various properties on our instance such as data, computed, watch, as well as best practices when using each one. Furthermore, we'll be looking at the various lifecycle hooks available within our Vue instance, allowing us to call particular functions at various stages of our application. Finally, we'll be investigating the Document Object Model (DOM) and why Vue implements a Virtual DOM for enhanced performance.
By the end of this chapter you will:
- Have a greater understanding of how this keyword works within JavaScript
- Understand how Vue proxies this keyword within Vue instances
- Use data properties to create reactive bindings
- Use computed properties to create declarative functions based on our data model
- Use watched properties to access asynchronous data and build upon the foundations of computed properties
- Use lifecycle hooks to activate functionality at particular stages of the Vue lifecycle
- Investigate the DOM and Virtual DOM for an understanding of how Vue renders data to the screen
To begin, let's start off by looking into how this works within JavaScript and how this relates to the context within our Vue instances.