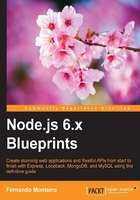
上QQ阅读APP看书,第一时间看更新
Protecting routes
At this point, we have enough code to configure secure access to our application. However, we still need to add a few more lines to the login and sign-up forms to make them work properly:
- Open
server/routes/index.js
and add the following lines after thelogin GET
route:/* POST login */ router.post('/login', passport.authenticate('local-login', { //Success go to Profile Page / Fail go to login page successRedirect : '/profile', failureRedirect : '/login', failureFlash : true }));
- Add these lines after the
signup GET
route:/* POST Signup */ router.post('/signup', passport.authenticate('local-signup', { //Success go to Profile Page / Fail go to Signup page successRedirect : '/profile', failureRedirect : '/signup', failureFlash : true }));
- Now let's add a simple function to check whether the user is logged in; at the end of
server/routes/index.js
, add the following code:/* check if user is logged in */ function isLoggedIn(req, res, next) { if (req.isAuthenticated()) return next(); res.redirect('/login'); }
- Let's add a simple route to a logout function and add the following code after the
isLoggedIn()
function:/* GET Logout Page */ router.get('/logout', function(req, res) { req.logout(); res.redirect('/'); });
- The last change is to add
isloggedin()
as a second parameter to the profile route. Add the following highlighted code:/* GET Profile page. */ router.get('/profile', isLoggedIn, function(req, res, next) { res.render('profile', { title: 'Profile Page', user : req.user, avatar: gravatar.url(req.user.email , {s: '100', r: 'x', d:'retro'}, true) }); });
The final index.js
file will look like this:
var express = require('express'); var router = express.Router(); var passport = require('passport'); // get gravatar icon from email var gravatar = require('gravatar'); /* GET home page. */ router.get('/', function(req, res, next) { res.render('index', { title: 'Express from server folder' }); }); /* GET login page. */ router.get('/login', function(req, res, next) { res.render('login', { title: 'Login Page', message: req.flash('loginMessage') }); }); /* POST login */ router.post('/login', passport.authenticate('local-login', { //Success go to Profile Page / Fail go to login page successRedirect : '/profile', failureRedirect : '/login', failureFlash : true })); /* GET Signup */ router.get('/signup', function(req, res) { res.render('signup', { title: 'Signup Page', message: req.flash('signupMessage') }); }); /* POST Signup */ router.post('/signup', passport.authenticate('local-signup', { //Success go to Profile Page / Fail go to Signup page successRedirect : '/profile', failureRedirect : '/signup', failureFlash : true })); /* GET Profile page. */ router.get('/profile', isLoggedIn, function(req, res, next) { res.render('profile', { title: 'Profile Page', user : req.user, avatar: gravatar.url(req.user.email , {s: '100', r: 'x', d: 'retro'}, true) }); }); /* check if user is logged in */ function isLoggedIn(req, res, next) { if (req.isAuthenticated()) return next(); res.redirect('/login'); } /* GET Logout Page */ router.get('/logout', function(req, res) { req.logout(); res.redirect('/'); }); module.exports = router;
We have almost everything set to finalize the application, but we still need to create a page for comments.