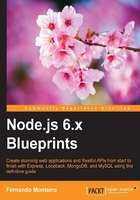
上QQ阅读APP看书,第一时间看更新
Creating the controllers folder
Instead of using the routes
folder to create the route and functions of the comments file, we will use another format and create the controllers
folder, where we can separate the route and the controller function, thus having a better modularization:
- Create a folder called
controllers
. - Create a file called
comments.js
and add the following code:// get gravatar icon from email var gravatar = require('gravatar'); // get Comments model var Comments = require('../models/comments'); // List Comments exports.list = function(req, res) { // List all comments and sort by Date Comments.find().sort('-created').populate('user', 'local.email').exec(function(error, comments) { if (error) { return res.send(400, { message: error }); } // Render result res.render('comments', { title: 'Comments Page', comments: comments, gravatar: gravatar.url(comments.email, {s: '80', r: 'x', d: 'retro'}, true) }); }); }; // Create Comments exports.create = function(req, res) { // create a new instance of the Comments model with request body var comments = new Comments(req.body); // Set current user (id) comments.user = req.user; // save the data received comments.save(function(error) { if (error) { return res.send(400, { message: error }); } // Redirect to comments res.redirect('/comments'); }); }; // Comments authorization middleware exports.hasAuthorization = function(req, res, next) { if (req.isAuthenticated()) return next(); res.redirect('/login'); };
- Let's import the controllers on the
app.js
file; add the following lines aftervar users = require('./server/routes/users')
:// Import comments controller var comments = require('./server/controllers/comments');
- Now add the comments route after
app.use('/users', users)
:// Setup routes for comments app.get('/comments', comments.hasAuthorization, comments.list); app.post('/comments', comments.hasAuthorization, comments.create);
- Create a file called
comments.ejs
atserver/pages
and add the following lines:<!DOCTYPE html> <html> <head> <title><%= title %></title> <% include ../partials/stylesheet %> </head> <body> <% include ../partials/header %> <div class="container"> <div class="row"> <div class="col-lg-6"> <h4 class="text-muted">Comments</h4> </div> <div class="col-lg-6"> <button type="button" class="btn btn-secondary pull-right" data-toggle="modal" data-target="#createPost"> Create Comment </button> </div> </div> <!-- Modal --> <div class="modal fade" id="createPost" tabindex="-1" role="dialog" aria-labelledby="myModalLabel" aria-hidden="true"> <div class="modal-dialog" role="document"> <div class="modal-content"> <form action="/comments" method="post"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-label="Close"> <span aria-hidden="true">×</span> </button> <h4 class="modal-title" id="myModalLabel"> Create Comment</h4> </div> <div class="modal-body"> <fieldset class="form-group"> <label for="inputitle">Title</label> <input type="text" id="inputitle" name="title" class="form-control" placeholder= "Comment Title" required=""> </fieldset> <fieldset class="form-group"> <label for="inputContent">Content</label> <textarea id="inputContent" name="content" rows="8" cols="40" class="form-control" placeholder="Comment Description" required=""> </textarea> </fieldset> </div> <div class="modal-footer"> <button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button> <button type="submit" class="btn btn-primary"> Save changes</button> </div> </form> </div> </div> </div> <hr> <div class="lead"> <div class="list-group"> <% comments.forEach(function(comments){ %> <a href="#" class="list-group-item"> <img src="<%= gravatar %>" alt="" style="float: left; margin-right: 10px"> <div class="comments"> <h4 class="list-group-item-heading"> <%= comments.title %></h4> <p class="list-group-item-text"> <%= comments.content %></p> <small class="text-muted">By: <%= comments.user.local.email %> </small> </div> </a> <% }); %> </div> </div> </div> <% include ../partials/footer %> <% include ../partials/javascript %> </body> </html>
- Note that we are using a simple Modal component from Twitter-bootstrap for the addition of comments, as shown in the following screenshot:
Model for the create comments screen
- The last step is to create a model for the comments; let's create a file named
comments.js
atserver/models/
and add the following code:// load the things we need var mongoose = require('mongoose'); var Schema = mongoose.Schema; var commentSchema = mongoose.Schema({ created: { type: Date, default: Date.now }, title: { type: String, default: '', trim: true, required: 'Title cannot be blank' }, content: { type: String, default: '', trim: true }, user: { type: Schema.ObjectId, ref: 'User' } }); module.exports = mongoose.model('Comments', commentSchema);