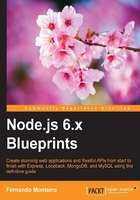
上QQ阅读APP看书,第一时间看更新
Creating a User scheme
With the help of Sequelize-cli
we will create a simple scheme for application users:
Open terminal/shell at the root project folder and type the following command:
sequelize model:create --name User --attributes "name:string, email:string"
You will see the following output on your terminal window:
Sequelize [Node: 6.3.0, CLI: 2.3.1, ORM: 3.19.3] Loaded configuration file "config/config.json". Using environment "development". Using gulpfile /usr/local/lib/node_modules/sequelize- cli/lib/gulpfile.js Starting 'model:create'... Finished 'model:create' after 13 ms
Let's check the user model file present at: models/User.js
, here add sequelize
using the define()
function to create the User scheme:
'use strict'; module.exports = function(sequelize, DataTypes) { var User = sequelize.define('User', { name: DataTypes.STRING, email: DataTypes.STRING }, { classMethods: { associate: function(models) { // associations can be defined here } } }); return User; };
Note that this command created the User.js
file within the models
folder and also created a migration file containing a hash and the name of the operation to be performed on the database within the migrations
folder.
This file contains the boilerplate necessary for creation of the User table in the database.
'use strict'; module.exports = { up: function(queryInterface, Sequelize) { return queryInterface.createTable('Users', { id: { allowNull: false, autoIncrement: true, primaryKey: true, type: Sequelize.INTEGER }, name: { type: Sequelize.STRING }, email: { type: Sequelize.STRING }, createdAt: { allowNull: false, type: Sequelize.DATE }, updatedAt: { allowNull: false, type: Sequelize.DATE } }); }, down: function(queryInterface, Sequelize) { return queryInterface.dropTable('Users'); } };