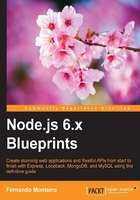
上QQ阅读APP看书,第一时间看更新
Creating Band schema
Let's create the schema that will store in the database the data of each band that the user creates in the system.
- Open terminal/shell and type the following command:
sequelize model:create --name Band --attributes "name:string, description:string, album:string, year:string, UserId:integer"
- As in the previous step, two files were created, one for migration of data and another to be used as a Band model, as the following code:
'use strict'; module.exports = function(sequelize, DataTypes) { var Band = sequelize.define('Band', { name: DataTypes.STRING, description: DataTypes.STRING, album: DataTypes.STRING, year: DataTypes.STRING, UserId: DataTypes.INTEGER }, { classMethods: { associate: function(models) { // associations can be defined here } } }); return Band; };
Creating associations between Band and User models
As the last step before using the schemes migration script, we will need to create the associations between the User model and the Band model. We will use the following associations:

Tip
You can find more about associations at the following link: http://docs.sequelizejs.com/en/latest/docs/associations/.
- Open the
User.js
model and add the following highlighted code:'use strict'; module.exports = function(sequelize, DataTypes) { var User = sequelize.define('User', { name: DataTypes.STRING, email: DataTypes.STRING }, { classMethods: { associate: function(models) { // associations can be defined here User.hasMany(models.Band); } } }); return User; };
- Open the
Band.js
model and add the following highlighted code:'use strict'; module.exports = function(sequelize, DataTypes) { var Band = sequelize.define('Band', { name: DataTypes.STRING, description: DataTypes.STRING, album: DataTypes.STRING, year: DataTypes.STRING, UserId: DataTypes.INTEGER }, { classMethods: { associate: function(models) { // associations can be defined here Band.belongsTo(models.User); } } }); return Band; };