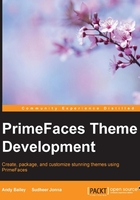
Creating the CurrentTheme Bean
Right-click on the session package, this time click on New…, and select the JSF Managed Bean option again.
Name the class as CurrentTheme
and change Scope to Session. The source code is once again opened automatically for us and it looks like this:
package com.andyba.pfthemes.session; */ import java.io.Serializable; import javax.inject.Named; import javax.enterprise.context.SessionScoped; /** * * @author Andy_2 */ @ManagedBean @SessionScoped public class CurrentTheme implements Serializable { /** * Creates a new instance of CurrentTheme */ public CurrentTheme() { } }
There is a new annotation, @SessionScoped
, which needs to be explained now:
@SessionScoped
: A session-scoped Bean is one that is created each time a user visits the application for the first time and lives for as long as the user session is valid. A session remains valid as long as the user actively uses the application. This way, we can use this Bean to keep track of information such as user preferences. Now, in a real-life application, we will store things such as user preferences in a database and have the user log in to our application so that we can read the preferences from the database.
The user preference that we are going to keep track of is the theme that the user chooses. We are now going to create a property for our Bean called theme
. In the Bean source code window, move the cursor to the line after the curly brace, as follows:
public CurrentTheme() { } // Cursor here
Press the Tab key once, right-click on the location where the cursor is, and select the Insert code… option. From the context menu that opens, select Add property…. On doing this, the following dialog box opens:

Change the name from string to theme. Then, add "aristo"
in the text field to the right of the = sign. We can leave the rest of the dialog box settings as they are and click on OK.
The following code is added to our Bean:
private String theme = "aristo"; /** * @return the theme */ public String getTheme() { return theme; } /** * @param theme the theme to set */ public void setTheme(String theme) { this.theme = theme; }
Remember that aristo
is the name of the default theme and we want to use this as the default user theme preference. Now, we need to tell the project how to use the Bean theme
property value to set the name of the theme being currently employed by the user.
The context
parameter that we used to set the bootstrap theme in the web.xml
project needs to be changed to access the CurrentTheme
Bean. Open the web.xml
file, locate the context
parameter entry that we added earlier, and change the parameter value according to the highlighted value in the following code:
<context-param>
<param-name>primefaces.THEME</param-name>
<param-value>#{currentTheme.theme}</param-value>
</context-param>
If you go back to the difference.xhtml
page, you will see that the name of the theme has been changed to #{currentTheme.theme}
, which isn't what we actually want. Change the last outputText
tag value attribute to the following:
value="#{currentTheme.theme}"
Refresh the page. Now, you will see that aristo
is the name of the current theme. Note that the theme has changed back to aristo as well.