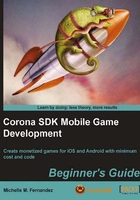
Building the application
Now that we have configured our application to landscape mode and set the display contents to scale on multiple devices, we're ready to start designing the game. Before we start writing some code for the game, we need to add in some art assets that will be displayed on the screen. You can find them in the Chapter 3 Resources
folder. You can download the project files accompanying this book from the Packt website. The following files that you'll need to add to your Breakout
project folder are as follows:
- alertBox.png
- bg.png
- mmScreen.png
- ball.png
- paddle.png
- brick.png
- playbtn.png
Displaying groups
An important function we'll be introducing in this game is display.newGroup()
. Groups allow you to add and remove child display objects. Initially, there are no children in a group. The local origin is at the parent object's origin; the reference point is initialised to this local origin. You can easily organize your display objects in separate groups and refer to them by their group name. For example, in Breakout, we'll combine menu items such as the Title screen and Play button in a group called menuScreenGroup
. Every time we access menuScreenGroup
, any display object defined by the group name will be called.
Working with system functions
The system functions we're going to introduce in this chapter will return information about the system (get device information, current orientation) and control system functions (enabling Multi-touch, controlling the idle time, Accelerometer, GPS). We'll be using the following system functions to return environment information on which our application will be running and the response frequency for accelerometer events.
This function returns information about the system on which the application is running.
Syntax: system.getInfo( param )
print( system.getInfo( "name" ) ) -- display the deviceID
Valid values for parameters are as follows:
"name"
—returns the name. For example, on the iTouch, this would be the name of the phone as it appears in iTunes, Pat's iTouch."model"
—returns the device type. These include:- "iPhone"
- "iPad"
- "iPhone Simulator"
- "Nexus One"
- "Droid"
- "myTouch"
- "Galaxy Tab"
"deviceID"
—returns the unique id of the device."environment"
—returns the environment that the app is running in. These include:"simulator"
: The Corona Simulator"device"
: iOS, Android device, and the Xcode Simulator
"platformName"
—returns the platform name (the OS name), that is, one of the following:- Mac OS X (Corona Simulator on Mac)
- Win (Corona Simulator on Windows))
- iPhone OS (all iOS devices))
- Android (all Android devices))
"platformVersion"
—returns a string representation of the platform version."version"
—returns the version of Corona used."build"
—returns the Corona build string."textureMemoryUsed"
—returns the texture memory usage in bytes."maxTextureSize"
—returns the maximum texture width or height supported by the device."architectureInfo"
—returns a string describing the underlying CPU architecture of the device you are running on.
This function sets the frequency of accelerometer events. The minimum frequency is 10 Hz and the maximum is 100 Hz on the iPhone. Accelerometer events are a significant drain on battery; so only increase the frequency when you need faster responses in games. Always try to lower the frequency whenever possible to conserve battery life.
Syntax: system.setAccelerometerInterval( frequency )
system.setAccelerometerInterval( 75 )
The function sets the sample interval in Hertz. Hertz is cycles per second, the number of measurements to take per second. If you set the frequency to 75, then the system will take 75 measurements per second.
After you have added the assets from the Chapter 3 Resources folder into your project folder, let's begin writing some code!