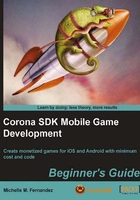
Time for action – creating variables for the game
For any application to start we'll need to make a main.lua
file. This has been discussed in the previous chapter when we worked with some sample code and ran it with the simulator.
The code will be structured accordingly in your main.lua
by the time the game is completed:
Necessary Classes
(For example: physics or ui)
Variables and Constants
Main Function
Object Methods
Call Main Function
(This always has to be called or your application will not run)
Formatting your code to make it look like the preceding structure is good practice on keeping things organized and running your application efficiently.
In this section, we'll be introducing the display group that will show the Main Menu screen and a Play button that the user will be able to interact with to move on to the Main Game screen. All in-game elements such as the paddle, ball, brick objects, and heads-up display elements follow after the player interacts with the Play button. We'll also be introducing win and lose conditions that will be referred to as the alertDisplayGroup
. All these game elements will be initialized in the beginning of our code.
- Create a new
main.lua
file in your text editor and save it to your project folder. - We're going to hide the status bar (specifically for iOS devices) and load the physics engine. Corona uses the Box2D engine that is already built into the SDK.
display.setStatusBar(display.HiddenStatusBar) local physics = require "physics" physics.start() physics.setGravity(0, 0) system.setAccelerometerInterval( 100 )
Note
More information on the Corona Physics API can be found on the Corona website at: http://developer.anscamobile.com/content/game-edition-box2d-physics-engine.
The Box2D physics engine used in Corona SDK was written by Erin Catto of Blizzard Entertainment. More information on Box2D can be found at: http://box2d.org/manual.pdf.
- Add in the menu screen objects.
local menuScreenGroup -- display.newGroup() local mmScreen local playBtn
- Add in the in-game screen objects.
local background local paddle local brick local ball
- Add in HUD elements for the score and level.
local scoreText local scoreNum local levelText local levelNum
- Next, we'll add in the alert display group for the win/lose conditions.
local alertDisplayGroup -- display.newGroup() local alertBox local conditionDisplay local messageText
- The following variables hold the values for the bricks display group, score, ball velocity, and in game events.
local _W = display.contentWidth / 2 local _H = display.contentHeight / 2 local bricks = display.newGroup() local brickWidth = 35 local brickHeight = 15 local row local column local score = 0 local scoreIncrease = 100 local currentLevel local vx = 3 local vy = -3 local gameEvent = ""
- Accelerometer events can only be tested on a device, so we're going to add a variable for touch events on the paddle by calling the
"simulator"
environment. This is so we can test the paddle movement in the Corona simulator. If you were to test the application on a device, the event listeners for touch and accelerometer on the paddle won't conflict.local isSimulator = "simulator" == system.getInfo("environment")
- Lastly, add in the
main()
function. This will start our application.function main() end --[[ This empty space will hold other functions and methods to run the application ]]-- main()
What just happened?
The display.setStatusBar(display.HiddenStatusBar)
is only applicable to iOS devices. It hides the appearance of the status bar.
A new Corona API we added to this game is the physics engine. We'll be adding physics parameters to the main game objects (paddle, ball, and bricks) for collision detection. Having setGravity(0,0)
will allow the ball to bounce throughout the playing field freely.
local menuScreenGroup
, local alertDisplayGroup
, and local bricks
are all forms of display groups we can separate and organize our display objects to. For example, local menuScreenGroup
is designated for the objects that show up on the Main Menu screen; that way they can be removed as a group and not as individual objects.
Some of the variables added already have values that are applied to certain game objects. There is already a set velocity for the ball using local vx = 3
and local vy = -3
. The x and y velocity determines how the ball moves on the game screen. Depending on the position the ball collides with an object, the ball will follow a continuous path. The brickWidth
and brickHeight
have a value that will stay constant throughout the application so we can line the brick objects evenly onscreen.
local gameEvent = " "
will store the game events such as "win"
, "lose"
, and "finished"
. When a function checks the game status for any of these events, it will display the proper condition onscreen.
We have added some system functions as well. We created local isSimulator = "simulator" == system.getInfo("environment")
so that it returns information about the system on which the application is running. This will be directed to the paddle touch events so that we can test the application in the simulator. If the build was to be ported on a device, you would only be able to use the accelerometer to move the paddle. The simulator can't test accelerometer events. The other system function is system.setAccelerometerInterval( 100 )
. It sets the frequency of accelerometer events. The minimum frequency is 10 Hz and the maximum is 100 Hz on the iPhone.
The empty function main()
set will start out the display hierarchy. Think of it as a storyboard. The first thing you see is an introduction and then some action happens in the middle that tells you about the main content. In this case, the main content is the gameplay. The last thing you see is some kind of ending or closure to tie the story altogether. The ending is the display of the win/lose conditions at the end of a level.