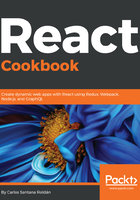
上QQ阅读APP看书,第一时间看更新
How to do it…
We will create a component where we will sum all the numbers entered in an input. We can take some of the last recipes to start from there:
- The first thing we will do is to modify our App.js and include the Numbers component:
import React, { Component } from 'react';
import Numbers from './Numbers/Numbers';
import Header from '../shared/components/layout/Header';
import Content from '../shared/components/layout/Content';
import Footer from '../shared/components/layout/Footer';
import './App.css';
class App extends Component {
render() {
return (
<div className="App">
<Header title="Understanding Pure Components" />
<Content>
<Numbers />
</Content>
<Footer />
</div>
);
}
}
export default App;
File: src/components/App.js
- Now we will create the Numbers component:
// Dependencies
import React, { Component } from 'react';
// Components
import Result from './Result';
// Styles
import './Numbers.css';
class Numbers extends Component {
state = {
numbers: '', // Here we will save the input value
results: [] // In this state we will save the results of the sums
};
handleNumberChange = e => {
const { target: { value } } = e;
// Converting the string value to array
// "12345" => ["1", "2", "3", "4", "5"]
const numbers = Array.from(value);
// Summing all numbers from the array
// ["1", "2", "3", "4", "5"] => 15
const result = numbers.reduce((a, b) => Number(a) + Number(b), 0);
// Updating the local state
this.setState({
numbers: value,
results: [...this.state.results, result]
});
}
render() {
return (
<div className="Numbers">
<input
type="number"
value={this.state.numbers}
onChange={this.handleNumberChange}
/>
{/* Rendering the results array */}
<ul>
{this.state.results.map((result, i) => (
<Result key={i} result={result} />
))}
</ul>
</div>
)
}
}
export default Numbers;
File: src/components/Numbers/Numbers.js
- Then, let's create the Result component (as a Class Component):
import React, { Component } from 'react';
class Result extends Component {
render() {
return <li>{this.props.result}</li>;
}
}
export default Result;
File: src/components/Numbers/Result.js
- Finally, the styles:
.Numbers {
padding: 30px;
}
.Numbers input[type=number]::-webkit-inner-spin-button,
.Numbers input[type=number]::-webkit-outer-spin-button {
-webkit-appearance: none;
margin: 0;
}
.Numbers input {
width: 500px;
height: 60px;
font-size: 20px;
outline: none;
border: 1px solid #ccc;
padding: 10px;
}
.Numbers ul {
margin: 0 auto;
padding: 0;
list-style: none;
width: 522px;
}
.Numbers ul li {
border-top: 1px solid #ccc;
border-left: 1px solid #ccc;
border-right: 1px solid #ccc;
padding: 10px;
}
.Numbers ul li:first-child {
border-top: none;
}
.Numbers ul li:last-child {
border-bottom: 1px solid #ccc;
}
File: src/components/Numbers/Numbers.css