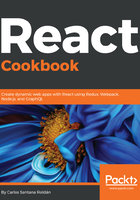
How it works…
If you run the application, you will see this:
As you can see, we are using an input with type number, which means we will only accept numbers if you start writing numbers (1, then 2, then 3, and such), you will see the results of the sum on each row (0 + 1 = 1, 1 + 2 = 3, 3 + 3 = 6).
Probably this looks very simple to you, but if let's inspect the application using React Developer Tools, we need to enable the Highlight Updates option.
After this, start writing multiple numbers in the input (quickly), and you will see all the renders that React is performing.
As you can see, React is doing a lot of renderings. When the highlights are red, it means the performance of that component is not good. Here's when Pure Components will help us; let's migrate our Result component to be a Pure Component:
import React, { PureComponent } from 'react';
class Result extends PureComponent {
render() {
return <li>{this.props.result}</li>;
}
}
export default Result;
Now if we try to do the same with the numbers, let's see the difference.
As you can see, with the Pure Component React, do less renders in comparison to a Class Component. Probably now you think that if we use a Stateless component instead of a Pure Component, the result will be the same. Unfortunately, this won't happen; if you want to verify this, let's change the Result component again and convert it into a Functional Component.:
import React from 'react';
const Result = props => <li>{props.result}</li>;
export default Result;
Even the code is less, but let's see what happen with the renders.
As you can see, the result is the same as the Class Component, which means not all the time using a Stateless component necessary will help us improve the performance of our application. If you have components that you consider are pure, consider converting them into Pure components.